c++stlmap用法
时间: 2023-12-28 16:16:51 浏览: 105
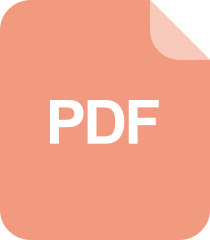
浅谈c++中的stl中的map用法详解

C++中的STL库提供了一个名为`std::map`的关联容器,它实现了一个有序键值对的集合。下面是`std::map`的用法示例:
```cpp
#include <iostream>
#include <map>
int main() {
// 创建一个空的std::map
std::map<int, std::string> employeeMap;
// 向map中添加元素
employeeMap.insert(std::make_pair(1, "Alice"));
employeeMap.insert(std::make_pair(2, "Bob"));
employeeMap.insert(std::make_pair(3, "Charlie"));
// 使用下标运算符访问元素
std::cout << "Employee with ID 2: " << employeeMap[2] << std::endl;
// 遍历map中的所有元素
for (const auto& pair : employeeMap) {
std::cout << "ID: " << pair.first << ", Name: " << pair.second << std::endl;
}
// 查找元素
auto it = employeeMap.find(3);
if (it != employeeMap.end()) {
std::cout << "Employee with ID 3 found: " << it->second << std::endl;
} else {
std::cout << "Employee with ID 3 not found." << std::endl;
}
// 删除元素
employeeMap.erase(1);
// 判断是否包含某个键
if (employeeMap.count(1) > 0) {
std::cout << "Employee with ID 1 is still in the map." << std::endl;
} else {
std::cout << "Employee with ID 1 is not in the map anymore." << std::endl;
}
return 0;
}
```
上述示例演示了如何创建一个`std::map`对象,添加元素,访问元素,遍历元素,查找元素,删除元素以及检查某个键是否存在的操作。你可以根据自己的需求进行适当的修改和扩展。
阅读全文
相关推荐
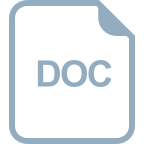


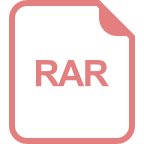
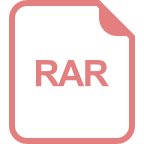
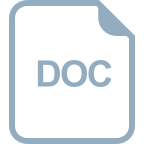
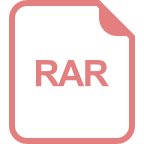
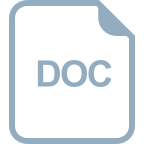
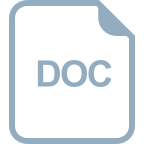
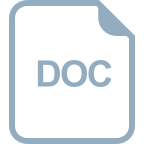
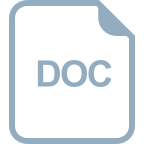
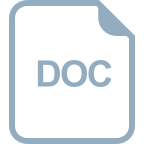
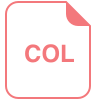
