在java当中将PDF转为word的详细代码
时间: 2024-10-15 07:09:12 浏览: 25
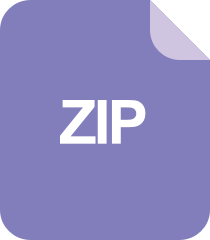
JAVA实现Linux中word转换pdf

在Java中将PDF转换为Word通常需要借助第三方库,如Apache POI(用于处理Microsoft Office文件,包括Word)和iText(一个流行的PDF库)。以下是一个简单的示例,展示如何使用这两个库结合来完成PDF到Word的转换:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.tool.xml.XMLWorkerHelper;
import org.apache.poi.xwpf.usermodel.*;
import org.apache.poi.xwpf.write.WriteFactory;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class PDFToWordConverter {
public static void main(String[] args) {
try {
// 读取PDF文件
PdfReader reader = new PdfReader("input.pdf");
// 创建Word文档
XWPFDocument document = new XWPFDocument();
// 循环处理每一页
for (int i = 1; i <= reader.getNumberOfPages(); i++) {
String text = extractTextFromPage(reader, i);
// 将文本添加到Word文档中
Paragraph paragraph = new Paragraph(text);
document.add(paragraph);
}
// 关闭PDF reader
reader.close();
// 写入Word文件
File outputWordFile = new File("output.docx");
WriteFactory factory = WriteFactory.create();
OutputStream out = new FileOutputStream(outputWordFile);
XMLWorkerHelper.getInstance().createWriter(out).write(document);
out.close();
System.out.println("PDF to Word conversion completed.");
} catch (IOException e) {
e.printStackTrace();
}
}
private static String extractTextFromPage(PdfReader reader, int page) throws IOException {
StringBuilder textBuilder = new StringBuilder();
reader.getPageContent(page).textFlattener().flatten(0, true, true);
char[] chars = reader.getTextCharacters();
int length = reader.getTextLength();
for (int i = 0; i < length; i++) {
textBuilder.append(chars[i]);
}
return textBuilder.toString();
}
}
```
这个代码首先打开PDF文件,然后逐页提取文本内容,再将其写入到一个新的Word文档中。注意实际操作中可能需要处理异常,并且需要在项目中引入相应的依赖。
阅读全文
相关推荐
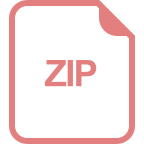
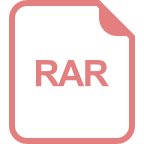


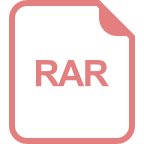
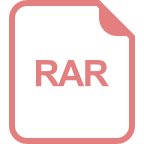


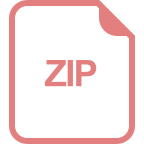
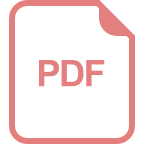
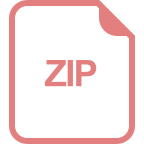
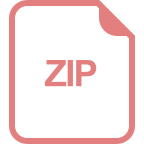
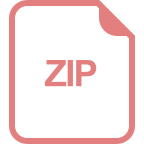
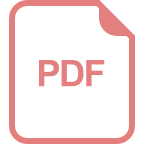
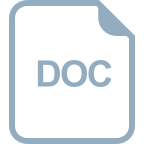


