c++实现hmacsha256加密算法
时间: 2023-07-29 17:14:27 浏览: 287
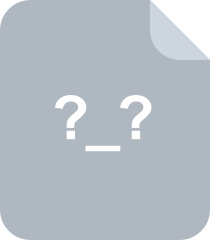
c++实现sha256
在C++中,可以使用Crypto++库来实现HMAC-SHA256加密算法。Crypto++是一个开源的加密库,提供了各种常见的加密算法实现。
以下是一个示例代码,演示如何使用Crypto++库来进行HMAC-SHA256加密:
```cpp
#include <iostream>
#include <string>
#include <cryptopp/cryptlib.h>
#include <cryptopp/hmac.h>
#include <cryptopp/sha.h>
#include <cryptopp/filters.h>
#include <cryptopp/hex.h>
std::string hmac_sha256(const std::string& key, const std::string& message) {
CryptoPP::HMAC<CryptoPP::SHA256> hmac((const byte*)key.data(), key.size());
std::string digest;
CryptoPP::StringSource(message, true,
new CryptoPP::HashFilter(hmac,
new CryptoPP::HexEncoder(new CryptoPP::StringSink(digest))));
return digest;
}
int main() {
std::string key = "secret_key";
std::string message = "Hello, World!";
std::string hmac = hmac_sha256(key, message);
std::cout << "HMAC-SHA256: " << hmac << std::endl;
return 0;
}
```
在上面的示例中,我们首先包含了Crypto++库的相关头文件。然后定义了一个名为`hmac_sha256`的函数,用于计算HMAC-SHA256值。该函数接受一个密钥和一个消息作为输入,并返回计算得到的HMAC-SHA256值。
在`main`函数中,我们指定了一个密钥和一个消息,然后调用`hmac_sha256`函数计算HMAC-SHA256值,并将结果打印输出。
需要注意的是,为了成功编译上述代码,需要先安装Crypto++库,并在编译时链接Crypto++库。具体的安装和链接方法可以参考Crypto++的官方文档或相关教程。
请确保在实际应用中使用安全的密钥和消息,并采取适当的安全措施来保护数据。
阅读全文
相关推荐
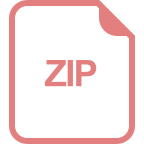
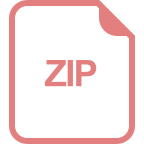
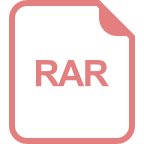
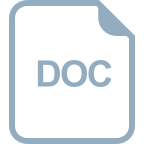

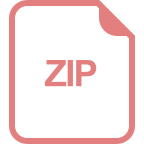
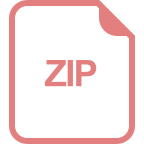
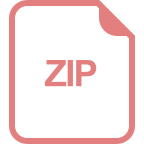
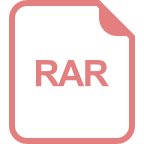
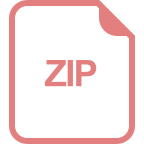
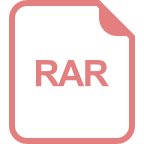
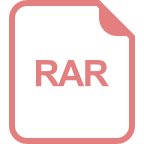
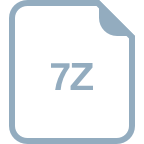
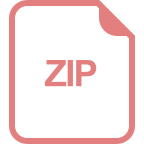
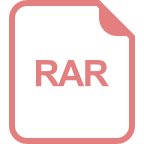
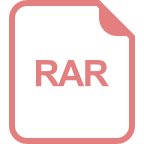
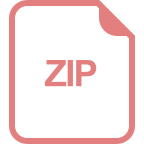