c#winfrom读写ini文件([set] User_ID=123 PassWord=MTIz [dev_qty] 点胶机=44 激光分板机=17 镭雕机=3)
时间: 2023-09-30 22:10:48 浏览: 68
你可以使用 `System.IO` 命名空间中的 `StreamReader` 和 `StreamWriter` 类来读写 INI 文件。下面是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
public class IniFile
{
private readonly string filePath;
private Dictionary<string, Dictionary<string, string>> sections;
public IniFile(string filePath)
{
this.filePath = filePath;
sections = new Dictionary<string, Dictionary<string, string>>();
ParseIniFile();
}
private void ParseIniFile()
{
string currentSection = null;
Dictionary<string, string> currentProperties = null;
foreach (string line in File.ReadLines(filePath))
{
if (line.StartsWith("[") && line.EndsWith("]"))
{
currentSection = line.Substring(1, line.Length - 2);
currentProperties = new Dictionary<string, string>();
sections[currentSection] = currentProperties;
}
else if (!string.IsNullOrWhiteSpace(line))
{
string[] parts = line.Split('=');
if (parts.Length == 2)
{
string key = parts[0].Trim();
string value = parts[1].Trim();
currentProperties[key] = value;
}
}
}
}
public string GetValue(string section, string key)
{
if (sections.TryGetValue(section, out var properties))
{
if (properties.TryGetValue(key, out var value))
{
return value;
}
}
return null;
}
public void SetValue(string section, string key, string value)
{
if (!sections.ContainsKey(section))
{
sections[section] = new Dictionary<string, string>();
}
sections[section][key] = value;
}
public void Save()
{
using (StreamWriter writer = new StreamWriter(filePath))
{
foreach (var section in sections)
{
writer.WriteLine($"[{section.Key}]");
foreach (var property in section.Value)
{
writer.WriteLine($"{property.Key}={property.Value}");
}
}
}
}
}
public class Program
{
public static void Main()
{
IniFile ini = new IniFile("path/to/your/file.ini");
// 读取值
string userID = ini.GetValue("set", "User_ID");
string password = ini.GetValue("set", "PassWord");
int glueMachineQty = int.Parse(ini.GetValue("dev_qty", "点胶机"));
Console.WriteLine($"User ID: {userID}");
Console.WriteLine($"Password: {password}");
Console.WriteLine($"Glue Machine Qty: {glueMachineQty}");
// 设置新值
ini.SetValue("set", "User_ID", "456");
ini.SetValue("set", "PassWord", "NDU2");
ini.SetValue("dev_qty", "点胶机", "55");
// 保存更改
ini.Save();
}
}
```
将上述代码中的 `path/to/your/file.ini` 替换为你的 INI 文件的路径。这个示例代码可以读取 INI 文件中的值,并且可以设置新的值并保存更改。
相关推荐
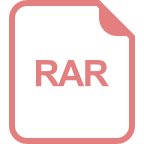














