如何在OkHttp拦截器中处理错误情况?
时间: 2024-09-28 08:15:05 浏览: 50
在OkHttp库中,你可以通过实现`Interceptor`接口并覆盖其`intercept()`方法来添加网络请求的拦截功能,包括处理错误情况。以下是基本步骤:
1. 创建一个自定义的拦截器类,继承`Interceptor`:
```java
import okhttp3.Interceptor;
import okhttp3.Request;
import okhttp3.Response;
public class CustomInterceptor implements Interceptor {
@Override
public Response intercept(Chain chain) throws IOException {
Request originalRequest = chain.request();
// 在这里添加错误处理逻辑,比如检查请求是否合法、缓存控制等
try {
// 对原始请求做修改,如添加头部信息
Request request = ...;
return chain.proceed(request);
} catch (Exception e) {
// 如果发生错误,可以创建一个ErrorResponse并返回
return newErrorResponse(e, originalRequest);
}
}
private Response newErrorResponse(Exception e, Request originalRequest) {
// 创建一个包含错误信息的新Response对象
int code = 500; // 根据实际异常类型设置错误码
String message = "An error occurred"; // 错误描述
// 构造新的Response实例,通常会包含错误码、错误消息以及错误堆栈跟踪
return new Response.Builder()
.code(code)
.protocol(chain.connection().protocol())
.message(message)
.request(originalRequest)
.cause(e)
.build();
}
}
```
2. 将自定义拦截器注册到OkHttpClient上:
```java
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(new CustomInterceptor())
.build();
```
在这个过程中,如果`proceed()`方法抛出异常,就会被捕获并在`newErrorResponse()`方法中转换为一个响应返回给客户端。
阅读全文
相关推荐
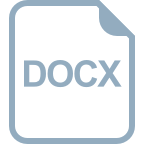
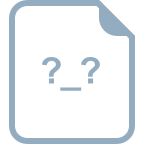
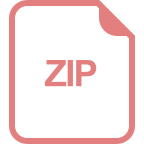
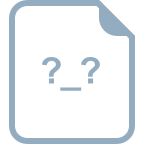
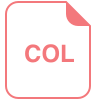
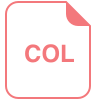

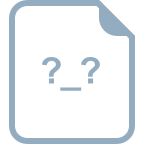
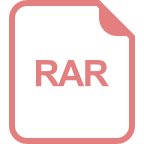
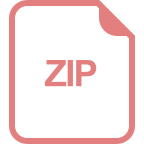
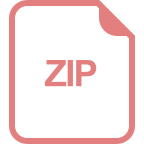
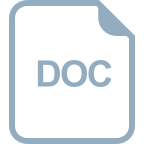
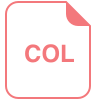
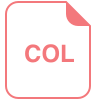
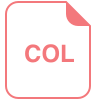



