c#与西门子通讯代码
时间: 2024-01-12 19:01:17 浏览: 27
"C"是拉丁字母表中的第3个字母。它在英语中有多种发音,如/k/音(例如"cat")、/s/音(例如"city")和/ʃ/音(例如"cheese")。此外,它还可以表示一些特定的概念和符号。例如,在数学中,"C"表示复数集;在化学中,它代表碳元素;在音乐中,它代表C大调;在计算机科学中,它代表C编程语言,是一种通用的编程语言;在电子商务领域,"C"也可以指代消费者。总之,"C"是一个常见且有多种用法和意义的字母。
相关问题
C# 西门子通讯代码
当然,我可以为您提供一些 C# 中使用西门子通讯的示例代码。以下是一个基本的示例,演示如何使用 S7-1200/1500 PLC 进行数据读写:
```C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
namespace SiemensCommunication
{
class Program
{
static void Main(string[] args)
{
// 定义需要连接的 PLC 的 IP 地址和端口号
string ipAddress = "192.168.0.1";
int port = 102;
try
{
// 创建 TCP 客户端套接字
TcpClient client = new TcpClient(ipAddress, port);
// 获取网络流对象
NetworkStream stream = client.GetStream();
// 定义用于发送和接收数据的字节数组
byte[] sendBuffer = new byte[256];
byte[] receiveBuffer = new byte[256];
// 执行一个读取操作(示例)
string readCommand = "Read Data Command";
sendBuffer = Encoding.ASCII.GetBytes(readCommand);
stream.Write(sendBuffer, 0, sendBuffer.Length);
// 接收来自 PLC 的响应
int bytesRead = stream.Read(receiveBuffer, 0, receiveBuffer.Length);
string response = Encoding.ASCII.GetString(receiveBuffer, 0, bytesRead);
Console.WriteLine("Received response from PLC: " + response);
// 关闭网络流和套接字
stream.Close();
client.Close();
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
Console.ReadLine();
}
}
}
```
请注意,这只是一个基本示例,实际应用中可能需要根据具体的通讯协议和 PLC 的要求进行一些调整。另外,您需要安装相关的库或 NuGet 包,例如 Siemens.SimaticS7.Net,以便在您的项目中使用西门子通讯。
C#与西门子PLC通讯
C#与西门子PLC通讯可以通过S7.net库来实现。S7.net是一个用于C#开发的西门子S7 PLC通信库,支持S7通信协议(TCP和ISO-over-TCP)和S7程序协议(读写PLC的数据块和符号表)。
具体的步骤如下:
1. 在Visual Studio中创建一个C#项目。
2. 在项目中添加S7.net库引用。
3. 在代码中实例化一个S7Client对象,设置PLC的IP地址和端口号。
4. 调用S7Client对象的Connect方法连接PLC。
5. 通过S7Client对象的Read方法读取PLC中的数据块或符号表。
6. 通过S7Client对象的Write方法写入数据到PLC中的数据块。
7. 调用S7Client对象的Disconnect方法断开与PLC的连接。
需要注意的是,PLC的数据块和符号表需要在PLC中进行配置和编写,才能在C#中进行读写操作。
相关推荐
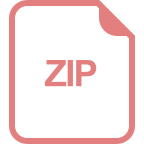
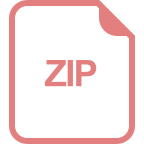












