去除url中的用户名和密码用C语言实现
时间: 2024-11-25 12:19:45 浏览: 6
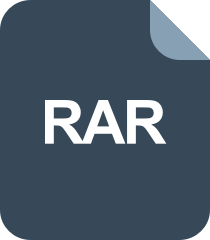
典型密码算法C语言实现_附录代码

在C语言中,处理URL并去除其中的用户名和密码通常需要先解析URL,这涉及到字符串操作和正则表达式。由于C语言标准库并没有内置的URL解析功能,我们可以使用一些第三方库如pcre(Perl兼容正则表达式库)或者第三方函数库如libcurl来帮助完成这个任务。
以下是一个简单的示例,使用`pcre`库来删除URL中的基本认证部分:
```c
#include <stdio.h>
#include <string.h>
#include <pcre.h>
void remove_credentials(char *url) {
size_t url_len = strlen(url);
pcre *pattern = pcre_compile("^(http|https)://([^:@]+):([^@]+)@", PCRE_DOTALL, NULL, &error_number, NULL);
if (error_number != 0) {
printf("Error compiling pattern: %d\n", error_number);
return;
}
char buffer[url_len];
strncpy(buffer, url, url_len); // Make a copy of the URL
int match_result = pcre_exec(pattern, NULL, buffer, url_len, 0, 0, NULL, 0);
if (match_result == 0 || match_result == PCRE_ERROR_NOMATCH) {
// No credentials found or invalid pattern
free(pattern);
printf("No credentials in the URL.\n");
} else {
char *start = buffer + match_result; // Move after matched part
char *end = strchr(start, '/'); // Find end of scheme and authority
if (end) {
*(end - 1) = '\0'; // Truncate at '/'
printf("Modified URL: %s\n", buffer);
}
free(pattern);
}
}
int main() {
char url[] = "http://username:password@example.com/path";
remove_credentials(url);
return 0;
}
```
请注意,这个示例假设URL总是从`http://`或`https://`开始,并且包含冒号分隔的用户名和密码。实际应用中可能需要更复杂的处理,比如支持其他协议、查询参数等。
阅读全文
相关推荐
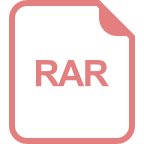















