编写一个c#程序:输入整数n (n大于等于0),分别利用for循环、while 循环、do...while循环求n!。↓
时间: 2024-09-25 14:09:14 浏览: 52
在C#中,计算阶乘(n!)通常涉及到一个循环结构,可以使用`for`、`while`和`do...while`三种方式进行。以下是分别使用这三种循环结构计算阶乘的示例程序:
### 1. 使用for循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1; // 初始化阶乘为1
for (int i = 1; i <= n; i++)
{
factorial *= i;
}
Console.WriteLine($"n! = {factorial}");
}
}
```
### 2. 使用while循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1;
int i = 1;
while (i <= n)
{
factorial *= i;
i++;
}
Console.WriteLine($"n! = {factorial}");
}
}
```
### 3. 使用do...while循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1;
int i = 1;
do
{
factorial *= i;
i++;
} while (i <= n);
Console.WriteLine($"n! = {factorial}");
}
}
```
在这三个例子中,都会检查给定的`n`是否大于0,然后计算从1到`n`的所有整数相乘的结果。
阅读全文
相关推荐
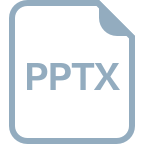
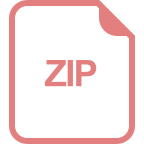
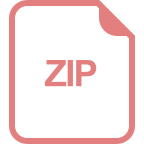




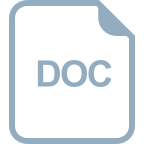










