3a效果爱心代码粒子c语言
时间: 2024-11-08 08:12:12 浏览: 84
在C语言中,实现3A效果(即平滑的爱心形状粒子效果)通常涉及图形处理库,如SDL、SFML等,但C标准库本身并不直接支持复杂的图形渲染。这类效果通常是通过循环绘制一系列小的爱心形像素点并更新它们的位置来模拟出来的。
下面是一个简单的伪代码示例,使用基本的位图操作来创建爱心粒子效果:
```c
#include <stdio.h>
#define SIZE 50 // 粒子大小
typedef struct {
int x, y; // 粒子位置
int dx, dy; // 加速度
} Particle;
void draw_heart_pixel(Particle p, int screen_width, int screen_height) {
int half_size = SIZE / 2;
int heart[] = { /* 画爱心的像素坐标数组 */ };
for (int i = -half_size; i <= half_size; ++i) {
int x = p.x + i * p.dx;
int y = p.y + i * p.dy;
if (x >= 0 && x < screen_width && y >= 0 && y < screen_height) {
putpixel(screen, x, y, heart[i]);
}
}
}
void update_particles(Particle* particles, int num_particles, int dt) {
for (int i = 0; i < num_particles; ++i) {
particles[i].x += particles[i].dx * dt;
particles[i].y += particles[i].dy * dt;
if (/* 边界条件处理 */) {
particles[i].dx *= -1; // 当碰到边界反弹
}
}
}
int main() {
Particle particles[] = {/* 初始化粒子位置和方向 */};
int screen_width, screen_height;
init_screen(&screen_width, &screen_height);
while (true) {
clear_screen(); // 清空屏幕
update_particles(particles, sizeof(particles) / sizeof(Particle), 1); // 更新粒子
for (int i = 0; i < sizeof(particles) / sizeof(Particle); ++i) {
draw_heart_pixel(particles[i], screen_width, screen_height);
}
update_frame(); // 显示新帧
}
return 0;
}
```
请注意,这个例子非常基础,实际的3D粒子系统会更复杂,涉及到颜色变化、速度调整等多个因素。而且,真正的爱心粒子效果一般在现代图形环境中使用,而不是简单的文本界面。如果你需要创建这样的效果,建议查阅相关的图形编程教程或使用专门的图形库来编写。
阅读全文
相关推荐
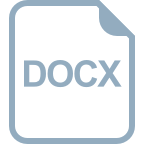
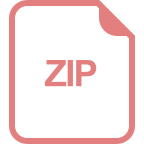
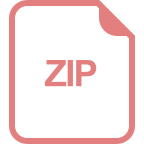















