如何在C#中将上传视频的文件路径存储为数据库字段?只写后端代码
时间: 2024-10-27 09:03:03 浏览: 19
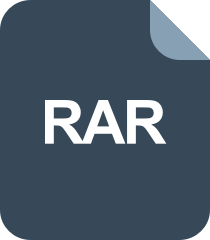
c# 操作文件上传数据库,再从数据库下载存放至本地

在C#中,为了将上传视频的文件路径存储到数据库字段,你需要经过几个步骤:
1. **连接数据库**:首先,确保已安装适当的数据库库,比如Entity Framework、ADO.NET等,并创建连接字符串。
```csharp
string connectionString = "Data Source=your_server;Initial Catalog=your_database;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
// ...
}
```
2. **获取文件路径**:用户上传视频后,保存文件到服务器,通常会有一个临时或持久化的存储路径。
```csharp
string filePath = Path.Combine(Server.MapPath("~/uploads"), "video.mp4");
```
3. **数据模型设计**:在数据库表中添加一个字段来存储视频路径,例如`VideoFilePath`,可以设置为`NVARCHAR`或`VARCHAR`类型的足够大以容纳视频路径。
4. **插入数据**:在事务上下文中,将文件路径插入到数据库。
```csharp
using (SqlCommand command = new SqlCommand("INSERT INTO YourTable (VideoFilePath) VALUES (@FilePath)", connection))
{
command.Parameters.AddWithValue("@FilePath", filePath);
await connection.OpenAsync();
int result = await command.ExecuteNonQueryAsync();
if (result > 0)
{
Console.WriteLine($"Inserted video path with ID {command.LastInsertedId}");
}
}
```
5. **错误处理**:别忘了处理可能出现的异常,如文件读取失败、网络问题或SQL错误。
阅读全文
相关推荐
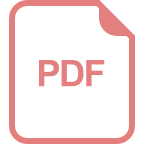
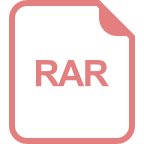
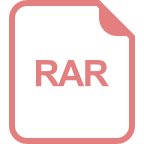
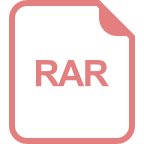
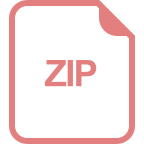
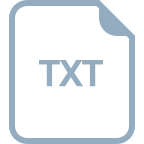
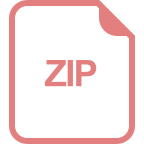
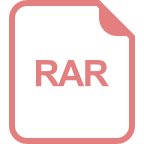
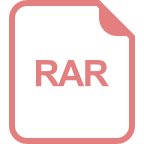
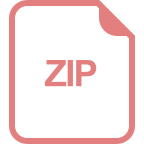
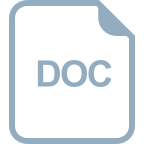
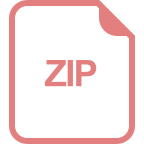
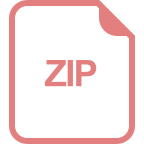
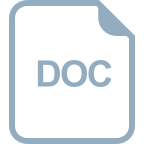
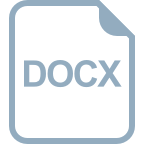