跨年烟花c++源代码
时间: 2024-12-04 19:15:05 浏览: 79
以下是一个使用C++编写的简单跨年烟花模拟程序。这个程序使用了基本的图形库来模拟烟花爆炸的效果。请注意,这个代码需要在你本地计算机上运行,并且可能需要安装一些图形库,如SFML或SDL。
```cpp
#include <SFML/Graphics.hpp>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <ctime>
class Particle {
public:
sf::CircleShape shape;
sf::Vector2f velocity;
float lifetime;
Particle(sf::Vector2f position) {
shape.setRadius(2.f);
shape.setPosition(position);
shape.setFillColor(sf::Color::White);
velocity = sf::Vector2f(std::rand() % 200 - 100, std::rand() % 200 - 100);
lifetime = 100;
}
void update() {
velocity.y += 5; // gravity
shape.move(velocity);
shape.setFillColor(sf::Color(255, 255, 255, --lifetime));
}
bool isAlive() const {
return lifetime > 0;
}
};
int main() {
std::srand(static_cast<unsigned>(std::time(nullptr)));
sf::RenderWindow window(sf::VideoMode(800, 600), "跨年烟花");
window.setFramerateLimit(60);
std::vector<Particle> particles;
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
if (event.type == sf::Event::MouseButtonPressed) {
for (int i = 0; i < 100; ++i)
particles.emplace_back(sf::Vector2f(event.mouseButton.x, event.mouseButton.y));
}
}
for (auto& particle : particles)
particle.update();
particles.erase(std::remove_if(particles.begin(), particles.end(),
[](const Particle& p) { return !p.isAlive(); }),
particles.end());
window.clear(sf::Color::Black);
for (const auto& particle : particles)
window.draw(particle.shape);
window.display();
}
return 0;
}
```
这个程序使用SFML库来创建一个窗口,并模拟烟花爆炸的效果。每次用户点击鼠标时,都会在点击位置生成100个粒子,模拟烟花爆炸的效果。
阅读全文
相关推荐
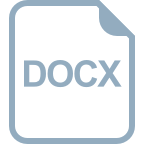
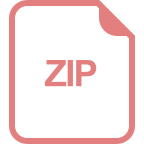
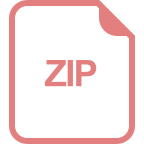
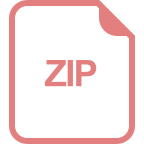
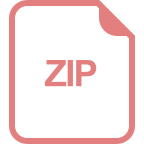
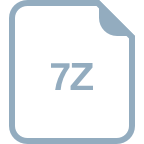
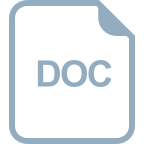
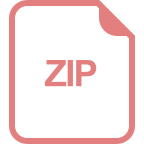
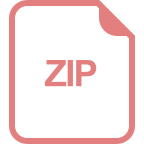
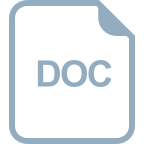
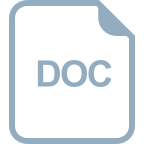
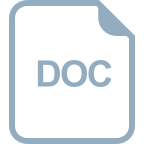
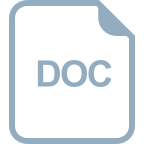