java class增加字段写法
时间: 2024-12-08 08:20:20 浏览: 10
在Java中,如果你想给一个已经存在的类增加一个新的字段(变量),你需要按照以下步骤操作:
1. **打开类文件**:首先找到并打开包含你要添加字段的那个类的源码文件。
2. **声明字段**:在类的合适位置(通常是public、protected、private权限,或者内部类中),使用数据类型加上字段名来定义新字段。例如:
```java
// 如果你想添加一个整型字段
private int newField;
// 或者一个字符串字段
String anotherField;
```
3. **考虑初始化**:如果你希望字段有默认值或者需要立即赋值,可以在定义时提供初始值,如 `private int newField = 0;`。
4. **遵守访问限制**:记住,Java的访问修饰符决定了其他类对这个字段的可见性和修改权限。如果你打算让其公开供外部使用,可以考虑使用public或protected;如果仅用于类内的数据处理,则选择private。
5. **编译类文件**:保存更改后,通过命令行或者IDE的构建工具编译Java类,确保没有语法错误。
相关问题
java定时器里面cros表达式写法
在Java的Timer或ScheduledExecutorService中,你可以使用cron表达式来设置任务的执行规则。Cron表达式是一种时间调度语言,它由6到7个字段组成,用于描述时间间隔。以下是cron表达式的常见格式:
1. 分钟 (0 - 59)
2. 小时 (0 - 23)
3. 日 (1 - 31)
4. 月份 (1 - 12 或 L-W for last week of month)
5. 星期 (0 - 7, where 0 or 7 is Sunday, 1 is Monday, etc.)
6. 年份 (1970-2199 或 * 对于所有年份)
例如,一个常见的cron表达式 "0 0 12 * * ?" 表示每天中午12点运行一次。
如果你需要在Java中使用cron表达式,可以使用`java.util.CronTrigger`配合`java.util.TimerTask`或`java.util.concurrent.Executors`中的`scheduleAtFixedRate()`等方法。确保导入`java.text.SimpleDateFormat`和`CronSequenceGenerator`库来解析和生成cron表达式。
```java
import java.util.*;
import java.text.*;
public class CronExample {
public static void main(String[] args) {
CronScheduleGenerator generator = new CronSequenceGenerator("0 0 12 * * ?");
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date());
long nextTime = generator.nextExecution(calendar.getTimeInMillis());
// 使用nextTime作为延迟参数启动任务
Timer timer = new Timer();
timer.schedule(new MyTask(), nextTime);
}
private static class MyTask extends TimerTask {
// ... 实现你需要的任务内容
}
}
```
在Swagger/OpenAPI规范中,paramType已经被弃用,改为了in字段,请用springboot项目中的java代码用注解的方式各自示例一下两种方式的完整写法
好的,下面分别给出paramType和in字段在Spring Boot项目中使用注解方式的示例:
1. paramType方式示例:
```java
@RestController
@RequestMapping("/api/v1/user")
public class UserController {
@GetMapping("/{userId}")
@ApiOperation(value = "获取用户信息", notes = "获取指定用户ID的用户信息")
@ApiImplicitParam(name = "userId", value = "用户ID", required = true, dataType = "long", paramType = "path")
public User getUserById(@PathVariable Long userId) {
// 通过用户ID获取用户信息
return userService.getUserById(userId);
}
}
```
在上面的示例中,使用了@ApiImplicitParam注解来描述参数,其中paramType字段被设置为path类型的参数。这样,Swagger就能够正确地生成对应的API文档。
2. in字段示例:
```java
@RestController
@RequestMapping("/api/v1/user")
public class UserController {
@GetMapping("/{userId}")
@ApiOperation(value = "获取用户信息", notes = "获取指定用户ID的用户信息")
@ApiImplicitParams({
@ApiImplicitParam(name = "userId", value = "用户ID", required = true, dataType = "long", paramType = "path")
})
public User getUserById(@PathVariable("userId") @ApiParam(value = "用户ID") Long userId) {
// 通过用户ID获取用户信息
return userService.getUserById(userId);
}
}
```
在上面的示例中,使用了@ApiImplicitParams和@ApiParam注解来描述参数,其中in字段被设置为path类型的参数。这样,Swagger就能够正确地生成对应的API文档。同时,我们还使用了@PathVariable注解来获取路径中的参数值。
阅读全文
相关推荐





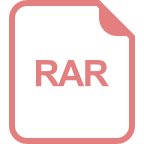










