C#创建一个缓存,通过线程定时器每隔10ms收集一次数据,缓存满了,或者1秒时间到了,将缓存中的数据写入到excel中的代码
时间: 2024-09-18 22:10:57 浏览: 49
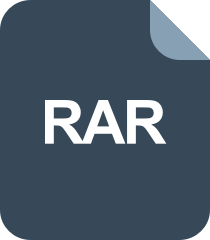
动态时钟(双缓存防止闪屏)c#语言编写

在C#中,你可以使用`MemoryCache`作为缓存,结合`System.Threading.Timer`进行定期数据收集,然后在达到一定条件时写入Excel。这里提供一个简化版的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Runtime.Caching;
using OfficeOpenXml;
class Program
{
private static MemoryCache cache = new MemoryCache("MyCache");
private const int MaxItemsInCache = 100; // 设置缓存最大容量
public static void Main()
{
// 定义线程定时器,每隔10毫秒收集数据
Timer timer = new Timer(CollectDataAndWriteToExcel, null, 0, 10); // 间隔10ms
// 启动定时器
timer.Change(10, Timeout.Infinite); // 每隔10ms触发,直到程序结束
Console.ReadLine();
}
private static void CollectDataAndWriteToExcel(object state)
{
try
{
// 检查缓存是否已满或过期
if (cache.Count >= MaxItemsInCache || DateTime.Now.Second % 60 == 0) // 每分钟检查一次
{
Dictionary<string, string> cachedData = cache.GetValues<object>("DataKey")?.Cast<string>().ToDictionary(k => k.Key, v => v.Value.ToString());
// 将缓存数据写入Excel
WriteToExcel(cachedData);
// 清空部分缓存,保持不超过最大容量
foreach (var item in cache)
{
if (item.Key != "DataKey" && cache[item.Key] is string) // 排除非字符串类型的缓存
cache.Remove(item.Key);
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
private static void WriteToExcel(Dictionary<string, string> data)
{
if (data.Any())
{
using var package = new ExcelPackage(new FileInfo("Output.xlsx"));
var sheet = package.Workbook.Worksheets.Add("Cache Data");
foreach (KeyValuePair<string, string> pair in data)
{
sheet.Cells[sheet.Dimension.End.Row++, 1].Value = pair.Value;
}
}
}
}
```
这个示例代码会每10毫秒检查缓存,如果缓存满了或者当前秒数是整数,就会清空部分数据并将剩余数据写入到Excel。注意这仅适用于缓存中的数据可以简单地转换为Excel单元格的内容。
阅读全文
相关推荐
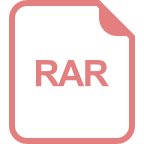
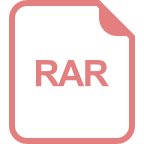


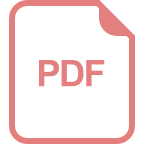
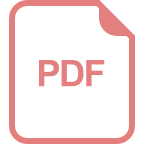
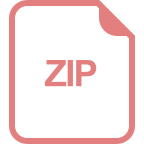
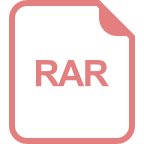
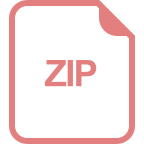
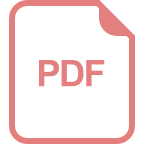
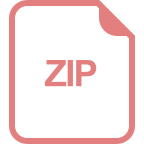
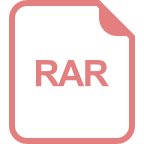
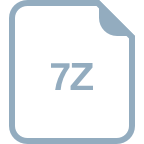
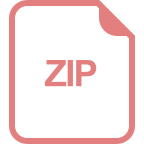
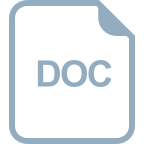
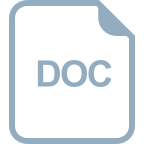
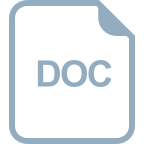
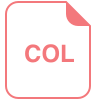