springboot 集成 python
时间: 2024-11-13 19:16:40 浏览: 24
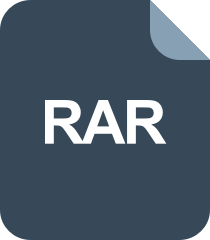
基于SpringBoot+Python多语言银行卡识别系统视频教程
Spring Boot 集成 Python 主要是通过第三方库来实现服务间的交互或数据处理。通常的做法是在 Spring Boot 应用中使用 RESTful API 来调用 Python 的 Web 服务,或者利用像 gunicorn 或 uWSGI 这样的工具将 Python 程序部署为 WSGI 服务器,然后通过 HTTP 请求与 Spring Boot 进行通信。
一种常见的做法是使用 `requests` 或者 `httpx` 这样的 Python HTTP 客户端库,从 Spring Boot 发送 GET、POST 等请求到 Python 服务,获取响应结果。另外,如果你需要在 Spring Boot 中执行 Python 脚本或者命令,可以借助 `subprocess` 模块。
另一方面,如果想让 Spring Boot 直接支持 Python,可以考虑使用 Jython(Python 的 Java 实现),但这并不常见,因为标准的 Java 和 Python 解释器之间有性能差异。
集成步骤大概如下:
1. 添加 Python 库依赖(如 requests)到 Spring Boot 项目的 pom.xml 文件中。
2. 创建 Python 服务并部署,提供一个稳定的 REST API 接口。
3. 在 Spring Boot 控制器中编写代码调用 Python 服务。
阅读全文
相关推荐
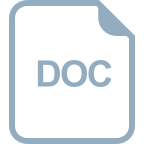
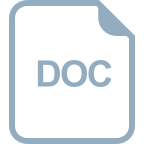
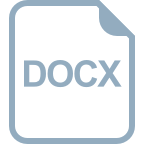
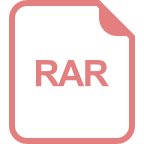
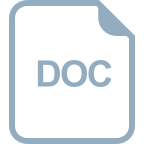

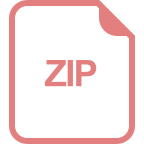
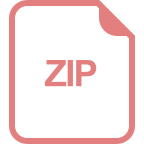
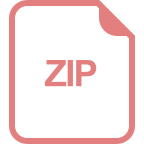
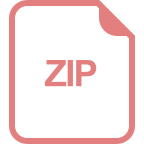
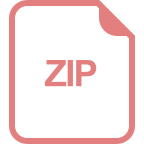
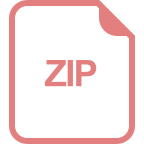
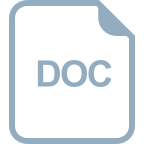
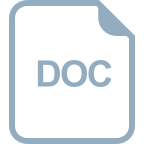
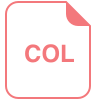


