如何在Spring Security中配置自动刷新令牌?
时间: 2024-08-13 13:06:52 浏览: 50
在Spring Security中,自动刷新令牌通常涉及到使用JWT(JSON Web Tokens)作为身份验证机制,以及一个适当的刷新令牌策略。以下是一般的步骤:
1. 添加依赖:首先,在你的`pom.xml`或`build.gradle`文件中添加Spring Security JWT和相关库的依赖。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
</dependency>
<!-- Gradle (Gradle Plugin) -->
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'io.jsonwebtoken:jjwt-api'
implementation 'io.jsonwebtoken:jjwt-impl:0.9.1' // 实际版本可能根据项目需求不同
```
2. 配置JWT:创建一个JWTTokenProvider,并在SecurityConfig中设置JWT相关的属性,如过期时间(access_token过期时间和refresh_token过期时间)和签发者。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
@Bean
public JwtAuthenticationProvider jwtAuthenticationProvider() {
JwtAuthenticationProvider provider = new JwtAuthenticationProvider(jwtTokenProvider);
provider.setJwtIdClaimName("sub");
return provider;
}
@Bean
public JwtTokenProvider jwtTokenProvider() {
return new JwtTokenProvider();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// 更多的Security配置...
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/auth/**").permitAll()
.anyRequest().authenticated()
.and()
.httpBasic().disable()
.cors().disable()
.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
@Bean
public FilterRegistrationBean jwtAuthenticationFilter() {
JwtAuthenticationFilter filter = new JwtAuthenticationFilter(authenticationManager());
filter.setTokenEnhancer(tokenEnhancer());
return new FilterRegistrationBean<>(filter);
}
private TokenEnhancer tokenEnhancer() {
return accessToken -> {
// 在这里处理token刷新逻辑,如果需要自动刷新,可以在适当时候创建新的refresh_token
// 如果刷新令牌可用并且未过期,返回新的access_token
// ...
return accessToken;
};
}
}
```
3. 刷新令牌策略:在`tokenEnhancer()`方法中,你可以检查当前的refresh_token是否有效并可以用来获取新的access_token。你可以选择一个策略,比如定期刷新(基于时间)或在access_token用尽之前刷新。
4. 使用刷新令牌:在API调用中,当用户访问受保护的资源且他们的access_token已过期时,后端服务器会检查是否有有效的refresh_token。如果有,将使用refresh_token通过JWT服务生成新的access_token并更新客户端的token缓存。
相关问题:
1. Spring Security中的JWT刷新令牌是如何工作的?
2. 如何在`tokenEnhancer()`中实现自动刷新策略?
3. 刷新令牌的存储通常在哪里?
相关推荐
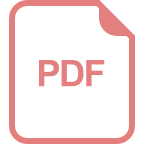
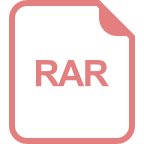
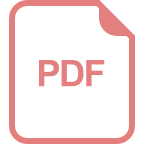














