python输入一个字符串,输出其中只出现了一次的字符及其下标。
时间: 2024-09-29 12:15:57 浏览: 42
在Python中,你可以通过创建一个字典来跟踪每个字符及其出现的次数。遍历字符串,对于每个字符,如果它还没在字典中,则添加到字典并设置计数为1,同时记录其索引;如果已经在字典中,增加计数。最后找出计数为1的字符及其索引。
下面是一个简单的示例代码:
```python
def find_unique_chars_and_indices(s):
char_dict = {}
unique_chars = []
for i, char in enumerate(s):
if char not in char_dict:
char_dict[char] = {'count': 1, 'indices': [i]}
else:
char_dict[char]['count'] += 1
for key, value in char_dict.items():
if value['count'] == 1:
unique_chars.append((key, value['indices'][0]))
return unique_chars
# 测试例子
input_str = "hello world"
result = find_unique_chars_and_indices(input_str)
print("只出现一次的字符及它们的下标:", result)
相关问题
python输入一个字符,输出其中只出现了一次的字符及其下标
在Python中,你可以通过字典数据结构来跟踪每个字符及其出现次数。遍历输入字符串时,如果一个字符第一次出现,我们可以将其添加到字典并记录其下标,如果再次出现则更新计数。以下是一个简单的示例函数:
```python
def find_unique_chars_and_indices(s):
char_dict = {}
indices = {}
for i, char in enumerate(s):
if char not in char_dict:
char_dict[char] = 1
indices[char] = [i]
else:
char_dict[char] += 1
indices[char].append(i)
unique_chars = {char: idxs for char, idxs in indices.items() if len(idxs) == 1}
return unique_chars
# 使用示例
input_str = input("请输入一个字符串: ")
result = find_unique_chars_and_indices(input_str)
print(f"只出现一次的字符及下标:{result}")
Python输入一个字符串,输出其中只出现了一次的字符及其下标。
可以使用Python的字典来解决这个问题。
首先,我们可以遍历字符串,并使用字典来记录每个字符出现的次数和下标。然后,再遍历一次字典,找到只出现了一次的字符及其下标。
以下是一个实现的示例代码:
```python
def find_unique_chars(string):
char_count = {} # 用于记录字符出现次数和下标的字典
for index, char in enumerate(string):
if char in char_count:
char_count[char][0] += 1
else:
char_count[char] = [1, index]
unique_chars = []
for char, count_index in char_count.items():
if count_index[0] == 1:
unique_chars.append((char, count_index[1]))
return unique_chars
# 例子输入
input_string = "abccdeffg"
result = find_unique_chars(input_string)
print(result)
```
输出结果为:
```
[('a', 0), ('b', 1), ('d', 4), ('g', 7)]
```
阅读全文
相关推荐
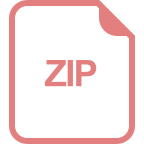
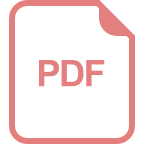
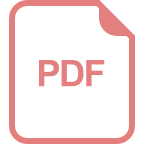













