html获取表格单元格和相邻单元格中间位置的js代码
时间: 2024-11-27 15:14:09 浏览: 4
在HTML中,你可以使用JavaScript配合DOM操作来获取表格单元格及其相邻单元格的位置。假设你想获取一个指定单元格`<td>`元素以及它上下左右相邻单元格的中心点坐标,可以这样做:
```javascript
// 假设tableId是你表格的ID,cellIndex是你想要获取的单元格索引(从0开始)
function getCellAndNeighbors(tableId, cellIndex) {
// 获取表格元素
var table = document.getElementById(tableId);
// 获取指定单元格
var cell = table.rows[cellIndex].cells[cellIndex];
// 定义相邻单元格的索引
var topCellIndex = cellIndex - 1;
var bottomCellIndex = cellIndex + 1;
var leftCellIndex = cell.cellIndex - 1;
var rightCellIndex = cell.cellIndex + 1;
// 确保索引在有效范围内
if (topCellIndex < 0 || bottomCellIndex >= table.rows.length || leftCellIndex < 0 || rightCellIndex >= table.columns) {
console.log('某个邻近单元格不存在');
return;
}
// 获取相邻单元格
var topCell = topCellIndex >= 0 ? table.rows[topCellIndex].cells[cellIndex] : null;
var bottomCell = bottomCellIndex < table.rows.length ? table.rows[bottomCellIndex].cells[cellIndex] : null;
var leftCell = leftCellIndex >= 0 ? table.rows[cellIndex].cells[leftCellIndex] : null;
var rightCell = rightCellIndex < table.columns ? table.rows[cellIndex].cells[rightCellIndex] : null;
// 计算每个单元格的中心位置
var cellCenterX = cell.offsetLeft + cell.offsetWidth / 2;
var cellCenterY = cell.offsetTop + cell.offsetHeight / 2;
var topCellCenterX = topCell ? topCell.offsetLeft + topCell.offsetWidth / 2 : undefined;
var topCellCenterY = topCell ? topCell.offsetTop + topCell.offsetHeight / 2 : undefined;
var bottomCellCenterX = bottomCell ? bottomCell.offsetLeft + bottomCell.offsetWidth / 2 : undefined;
var bottomCellCenterY = bottomCell ? bottomCell.offsetTop + bottomCell.offsetHeight / 2 : undefined;
var leftCellCenterX = leftCell ? leftCell.offsetLeft + leftCell.offsetWidth / 2 : undefined;
var leftCellCenterY = leftCell ? leftCell.offsetTop + leftCell.offsetHeight / 2 : undefined;
var rightCellCenterX = rightCell ? rightCell.offsetLeft + rightCell.offsetWidth / 2 : undefined;
var rightCellCenterY = rightCell ? rightCell.offsetTop + rightCell.offsetHeight / 2 : undefined;
// 返回包含所有信息的对象
return {
cell: { x: cellCenterX, y: cellCenterY },
top: { x: topCellCenterX, y: topCellCenterY },
bottom: { x: bottomCellCenterX, y: bottomCellCenterY },
left: { x: leftCellCenterX, y: leftCellCenterY },
right: { x: rightCellCenterX, y: rightCellCenterY }
};
}
// 调用函数并打印结果
var result = getCellAndNeighbors('yourTableId', 2); // 传入你的表格ID和单元格索引
console.log(result);
```
阅读全文
相关推荐
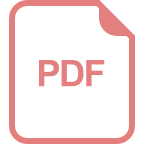
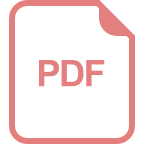
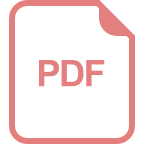















