uart串口通信c语言实现
时间: 2023-09-09 19:11:50 浏览: 225
UART串口通信在C语言中的实现可以通过使用相应的串口库来实现。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd;
char data[255];
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd == -1) {
perror("打开串口失败");
return -1;
}
// 配置串口参数
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL;
options.c_cflag |= CREAD;
tcsetattr(fd, TCSANOW, &options);
// 读取串口数据
memset(data, 0, sizeof(data));
int len = read(fd, data, sizeof(data));
if (len == -1) {
perror("读取串口数据失败");
return -1;
}
printf("接收到的数据:%s\n", data);
// 关闭串口设备
close(fd);
return 0;
}
```
在这个示例中,我们首先使用`open`函数打开了串口设备`/dev/ttyS0`,并指定了读写权限。然后,使用`tcgetattr`函数获取当前串口的配置参数,并使用`cfsetispeed`和`cfsetospeed`函数设置波特率为9600bps。接下来,我们将`CLOCAL`和`CREAD`标志位设置为1,表示本地连接和可读。然后使用`tcsetattr`函数将新的配置参数应用到串口设备上。
之后,我们使用`read`函数从串口读取数据,读取的数据存储在`data`数组中。通过输出来验证是否成功接收到了数据。最后,我们使用`close`函数关闭串口设备。
请注意,此示例仅用于演示目的,实际使用中可能需要根据具体情况进行适当的修改。
阅读全文
相关推荐
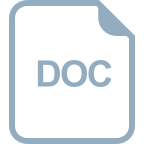
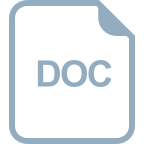
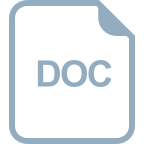
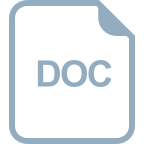
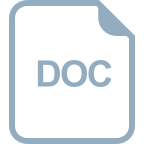
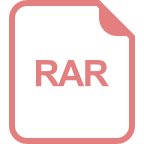
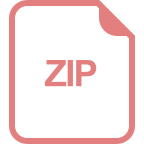
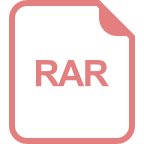
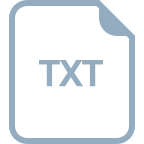
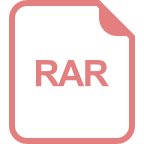
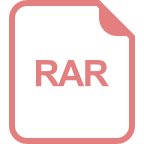
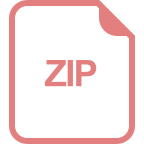
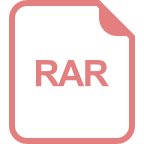
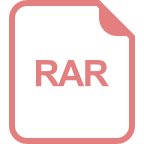
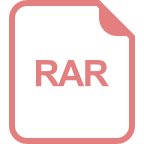
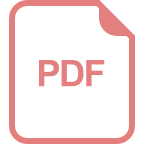
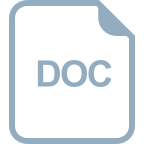
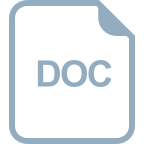
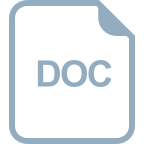