rust中的pow算法的定义
时间: 2024-09-28 12:10:00 浏览: 68
在 Rust 中,没有内置的 `pow` 函数专门用于计算幂运算,就像 C 或 Python 那样。但是你可以使用 `std::ops` trait 中的 `pow` 方法来自行实现这个操作,如果你需要自定义的行为,比如大数乘法或者特定精度的计算。
```rust
use std::ops::{FnOnce, Pow};
// 自定义一个 pow 关联函数
trait MyMath: FnOnce(f64, f64) -> f64 {
fn pow(self, base: f64, exponent: f64) -> f64;
}
impl MyMath for f64 {
// 使用浮点数的内置 pow 函数
fn pow(self, exponent: f64) -> f64 {
self.pow(exponent)
}
}
fn main() {
let result = 2.0.pow(3.0); // 这里会调用自定义的 pow 方法
println!("2 to the power of 3 is {}", result);
}
```
如果你想在库中提供一个通用的 pow 函数,可以创建一个闭包或者一个模块:
```rust
fn pow(base: f64, exponent: f64) -> f64 {
// 实现 pow 算法的代码...
}
fn custom_pow<T: MyMath>(base: T, exponent: f64) -> T {
base.pow(exponent)
}
let result = custom_pow(2.0, 3.0);
```
阅读全文
相关推荐
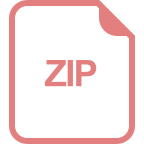
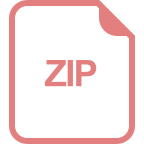
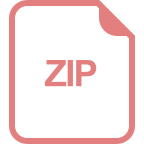
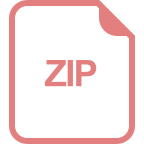
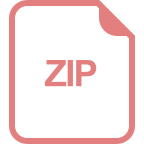
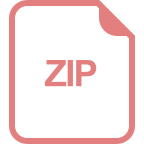
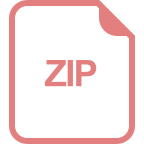
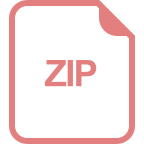
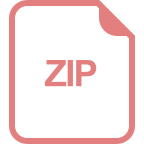
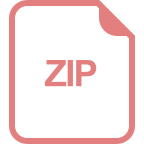
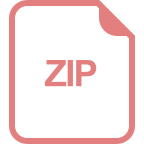
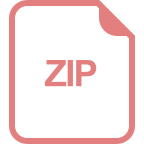
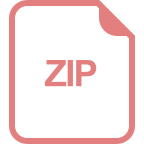
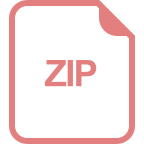
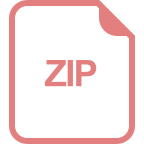
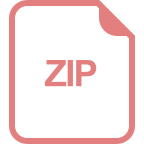