qt 使用FFmpeg播放视频
时间: 2024-12-08 20:12:11 浏览: 23
在Qt中使用FFmpeg播放视频可以通过以下步骤实现:
1. **下载和编译FFmpeg库**:
首先,你需要下载FFmpeg的源代码并编译生成所需的库文件。你可以从FFmpeg的官方网站下载源代码,并按照官方文档进行编译。
2. **配置Qt项目文件**:
在你的Qt项目的`.pro`文件中,添加FFmpeg的头文件和库文件路径。例如:
```plaintext
INCLUDEPATH += /path/to/ffmpeg/include
LIBS += -L/path/to/ffmpeg/lib -lavcodec -lavformat -lavutil -lswscale -lavdevice -lswresample
```
3. **创建视频播放器类**:
创建一个类来管理视频播放,包括初始化FFmpeg、解码视频帧、显示视频帧等。例如:
```cpp
#include <QWidget>
#include <QImage>
#include <QTimer>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
class VideoPlayer : public QWidget {
Q_OBJECT
public:
VideoPlayer(QWidget *parent = nullptr);
~VideoPlayer();
void open(const QString &filePath);
protected:
void paintEvent(QPaintEvent *event) override;
private slots:
void updateFrame();
private:
AVFormatContext *formatContext;
AVCodecContext *codecContext;
AVFrame *frame;
AVFrame *rgbFrame;
SwsContext *swsContext;
QImage image;
QTimer *timer;
};
```
4. **实现视频播放器类**:
在实现文件中,编写初始化、解码和显示视频帧的代码。例如:
```cpp
VideoPlayer::VideoPlayer(QWidget *parent) : QWidget(parent), formatContext(nullptr), codecContext(nullptr), frame(nullptr), rgbFrame(nullptr), swsContext(nullptr), timer(nullptr) {
av_register_all();
}
VideoPlayer::~VideoPlayer() {
if (timer) {
timer->stop();
}
if (rgbFrame) {
av_frame_free(&rgbFrame);
}
if (frame) {
av_frame_free(&frame);
}
if (codecContext) {
avcodec_free_context(&codecContext);
}
if (formatContext) {
avformat_close_input(&formatContext);
}
if (swsContext) {
sws_freeContext(swsContext);
}
}
void VideoPlayer::open(const QString &filePath) {
if (avformat_open_input(&formatContext, filePath.toUtf8().constData(), nullptr, nullptr) != 0) {
qDebug("Could not open file");
return;
}
if (avformat_find_stream_info(formatContext, nullptr) < 0) {
qDebug("Could not find stream information");
return;
}
AVCodec *codec = nullptr;
int videoStreamIndex = av_find_best_stream(formatContext, AVMEDIA_TYPE_VIDEO, -1, -1, &codec, 0);
if (videoStreamIndex < 0) {
qDebug("Could not find video stream");
return;
}
codecContext = avcodec_alloc_context3(codec);
if (!codecContext) {
qDebug("Could not allocate codec context");
return;
}
if (avcodec_parameters_to_context(codecContext, formatContext->streams[videoStreamIndex]->codecpar) < 0) {
qDebug("Could not copy codec parameters to codec context");
return;
}
if (avcodec_open2(codecContext, codec, nullptr) < 0) {
qDebug("Could not open codec");
return;
}
frame = av_frame_alloc();
rgbFrame = av_frame_alloc();
if (!frame || !rgbFrame) {
qDebug("Could not allocate frame");
return;
}
int numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB32, codecContext->width, codecContext->height, 1);
uint8_t *buffer = (uint8_t *)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(rgbFrame->data, rgbFrame->linesize, buffer, AV_PIX_FMT_RGB32, codecContext->width, codecContext->height, 1);
swsContext = sws_getContext(codecContext->width, codecContext->height, codecContext->pix_fmt, codecContext->width, codecContext->height, AV_PIX_FMT_RGB32, SWS_BILINEAR, nullptr, nullptr, nullptr);
timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &VideoPlayer::updateFrame);
timer->start(33);
}
void VideoPlayer::updateFrame() {
AVPacket packet;
while (av_read_frame(formatContext, &packet) >= 0) {
if (packet.stream_index == av_find_best_stream(formatContext, AVMEDIA_TYPE_VIDEO, -1, -1, nullptr, 0)) {
avcodec_send_packet(codecContext, &packet);
if (avcodec_receive_frame(codecContext, frame) == 0) {
sws_scale(swsContext, (uint8_t const * const *)frame->data, frame->linesize, 0, codecContext->height, rgbFrame->data, rgbFrame->linesize);
image = QImage(rgbFrame->data[0], codecContext->width, codecContext->height, QImage::Format_RGB32);
update();
}
}
av_packet_unref(&packet);
}
}
void VideoPlayer::paintEvent(QPaintEvent *event) {
QPainter painter(this);
painter.drawImage(0, 0, image);
}
```
5. **使用视频播放器类**:
在你的主窗口类中,创建一个`VideoPlayer`对象并调用`open`方法。例如:
```cpp
#include "VideoPlayer.h"
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
VideoPlayer *videoPlayer;
};
```
```cpp
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), videoPlayer(new VideoPlayer(this)) {
setCentralWidget(videoPlayer);
videoPlayer->open("path/to/video/file.mp4");
}
MainWindow::~MainWindow() {
delete videoPlayer;
}
```
阅读全文
相关推荐
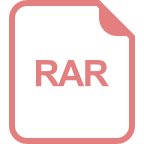
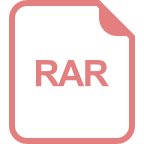
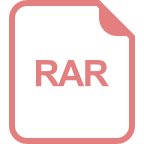

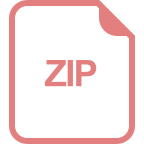
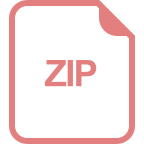
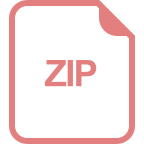
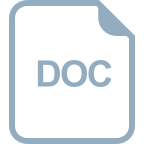







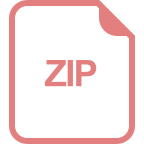
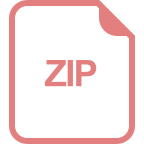
