plt.plot画一个矩形框
时间: 2023-11-16 07:54:34 浏览: 115
可以使用matplotlib库中的Rectangle类来画矩形框,具体代码如下:
```python
import matplotlib.pyplot as plt
from matplotlib.patches import Rectangle
fig, ax = plt.subplots()
rect = Rectangle((0.2, 0.3), 0.4, 0.5, linewidth=1, edgecolor='r', facecolor='none')
ax.add_patch(rect)
plt.show()
```
其中,Rectangle类的第一个参数是矩形框的左下角坐标,第二个参数是矩形框的宽度和高度,linewidth参数控制边框线条宽度,edgecolor参数控制边框颜色,facecolor参数控制填充颜色。
相关问题
np.meshgrid生成倾斜的矩形框
np.meshgrid是一个用于生成网格坐标的函数,它可以用来创建二维坐标矩阵。在生成倾斜的矩形框时,可以利用np.meshgrid生成两个坐标轴的网格矩阵,然后通过线性变换来实现倾斜效果。
下面是一个示例代码,演示如何使用np.meshgrid生成倾斜的矩形框:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义矩形框的宽度和高度
width = 5
height = 3
# 生成坐标轴的网格矩阵
x = np.linspace(0, width, num=100)
y = np.linspace(0, height, num=100)
X, Y = np.meshgrid(x, y)
# 定义线性变换矩阵
theta = np.radians(30) # 倾斜角度
T = np.array([[np.cos(theta), -np.sin(theta)],
[np.sin(theta), np.cos(theta)]])
# 进行线性变换
X_transformed, Y_transformed = np.dot(T, [X.flatten(), Y.flatten()])
# 将变换后的坐标重新恢复为矩阵形式
X_transformed = X_transformed.reshape(X.shape)
Y_transformed = Y_transformed.reshape(Y.shape)
# 绘制倾斜的矩形框
plt.figure()
plt.plot(X_transformed, Y_transformed, color='red')
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Skewed Rectangle')
plt.grid(True)
plt.axis('equal')
plt.show()
```
运行以上代码,将会生成一个倾斜的矩形框的图像。
t1 = time.time() # Note that compactness is defined differently because a grid is not used. Lower compactness for maskSLIC is equivalent segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=100, recompute_seeds=True, plot_examples=True, enforce_connectivity=True) print("Time: {:.2f} s".format(time.time() - t1)) for segment_id in np.unique(segments): mask = segments == segment_id masked_image = img.copy() masked_image[~mask] = 0 cv2.imwrite(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\segment_{}.png'.format(segment_id), masked_image) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=0.5) plt.axis('off') plt.savefig(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\maskslic.png')提取每个子块图像的位置和大小信息
可以使用 `regionprops` 函数从分割结果中提取每个子块的位置和大小信息。具体步骤如下:
1. 将分割结果转换为标签图像,其中每个子块的像素值为其对应的标签值。
2. 使用 `regionprops` 函数提取标签图像中每个区域的属性信息,包括区域的位置、大小、像素值等。
3. 遍历所有区域,获取其位置和大小信息。
下面是示例代码:
```python
from skimage.measure import label, regionprops
# 将分割结果转换为标签图像
label_img = label(segments)
# 使用 regionprops 函数提取标签图像中每个区域的属性信息
props = regionprops(label_img)
# 遍历所有区域,获取其位置和大小信息
for prop in props:
# 获取区域的标签值、位置和大小信息
label_value = prop.label
bbox = prop.bbox # (min_row, min_col, max_row, max_col)
height = bbox[2] - bbox[0]
width = bbox[3] - bbox[1]
# 在原图上绘制该区域的边界框
cv2.rectangle(img, (bbox[1], bbox[0]), (bbox[3], bbox[2]), (0, 255, 0), 2)
# 输出该区域的位置和大小信息
print("Label: {}, Position: ({}, {}), Size: {} x {}".format(label_value, bbox[1], bbox[0], width, height))
```
其中,`bbox` 表示区域的最小外接矩形,其格式为 `(min_row, min_col, max_row, max_col)`,分别表示矩形左上角和右下角的行列坐标。`height` 和 `width` 分别表示矩形的高度和宽度。在实际使用中,可以根据需求选择输出或保存这些信息。
阅读全文
相关推荐


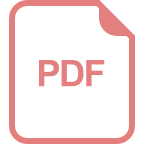
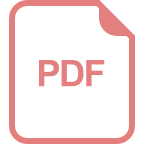
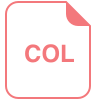
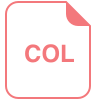
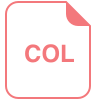






