c++定义一个时间类型Time,并进行求和
时间: 2025-01-01 18:39:56 浏览: 6
在C++中,我们可以创建一个自定义的时间类型`Time`来表示小时、分钟和秒。为了方便,我们通常使用结构体(struct)或者类(class)来封装这些属性。这里我会演示如何使用结构体来定义:
```cpp
#include <iostream>
using namespace std;
// 定义Time结构体
struct Time {
int hours;
int minutes;
int seconds;
};
// 定义求和函数,将两个Time对象相加
Time sumTimes(Time t1, Time t2) {
// 确保每个时间单位不超过最大值,防止溢出
if (t1.hours + t2.hours > 23) {
t1.hours = t1.hours % 24; // 将超过24小时的部分转移到天数上
}
if (t2.hours + t1.hours > 23) {
t2.hours = t2.hours % 24;
}
if (t1.minutes + t2.minutes > 59) {
t1.minutes = t1.minutes % 60;
}
if (t2.minutes + t1.minutes > 59) {
t2.minutes = t2.minutes % 60;
}
if (t1.seconds + t2.seconds > 59) {
t1.seconds = t1.seconds % 60;
}
if (t2.seconds + t1.seconds > 59) {
t2.seconds = t2.seconds % 60;
}
Time result;
result.hours = t1.hours + t2.hours;
result.minutes = t1.minutes + t2.minutes;
result.seconds = t1.seconds + t2.seconds;
return result;
}
int main() {
Time time1 = {3, 45, 10};
Time time2 = {9, 30, 0};
Time sum = sumTimes(time1, time2);
cout << "Sum of times is: " << sum.hours << ":" << sum.minutes << ":" << sum.seconds << endl;
return 0;
}
```
在这个例子中,`sumTimes`函数接收两个`Time`类型的参数,然后将它们的小时、分钟和秒分别累加,如果超出了范围就取余数。注意,这个简单的实现假设输入的时间不会超出一天24小时的限制。
阅读全文
相关推荐





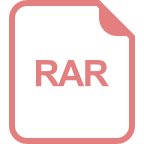












