将一个无序的单链表变为有序 c语言实现
时间: 2024-10-11 15:07:52 浏览: 11
在C语言中,将一个无序的单链表转换为有序通常会采用排序算法(如冒泡排序、插入排序或归并排序),然后通过修改原链表节点来达到目的。这里我们以插入排序为例,因为它的过程比较直观:
首先,我们需要定义一个链表结构体,包含数据域和指向下一个节点的指针:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
接着,我们可以编写一个函数来进行插入排序:
```c
void sortList(Node** head) {
if (*head == NULL || (*head)->next == NULL)
return; // 空链表或只有一个元素,已经是有序的
Node* current = *head;
Node* sorted = NULL;
while (current != NULL) {
Node* temp = current->next;
bool inserted = false;
// 插入当前节点到已排序部分的正确位置
while (sorted != NULL && sorted->data < current->data) {
sorted = sorted->next;
inserted = true;
}
if (inserted) {
current->next = sorted;
} else {
sorted = current;
}
current = temp;
}
*head = sorted; // 更新头结点为排序后的链表头
}
```
这个函数遍历链表,对每个节点找到其在已排序部分的正确位置,并插入到那里。最后返回的是一个新的有序链表,原始链表保持不变。
相关推荐
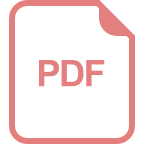
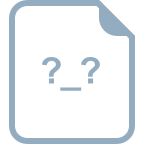
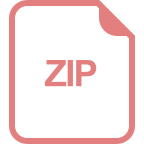














