c++读取rinex2.1文件的代码
时间: 2024-10-29 09:21:14 浏览: 46
在C++中读取RINEX(Receiver Independent Exchange Format)2.1格式的观测数据文件,通常需要使用文本文件流操作以及一些解析工具来处理文件中的特定结构,比如时间、坐标等信息。以下是一个简单的示例,展示了如何打开文件、逐行读取并解析基本的数据:
```cpp
#include <fstream>
#include <string>
#include <vector>
#include <sstream>
// 假设我们有一个名为`Observation`的结构体来存储 Rinex 数据
struct Observation {
double time;
double latitude;
double longitude;
// ... 其他字段
};
std::vector<Observation> readRinexFile(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("无法打开文件");
}
std::vector<Observation> observations;
for (std::string line; std::getline(file, line);) {
std::istringstream iss(line);
Observation obs;
// 假设第一列是时间,其他列按照顺序是纬度和经度
iss >> obs.time; // 根据实际文件格式调整
iss >> obs.latitude >> obs.longitude; // 同上
// 如果格式不正确,这里可以添加错误检查和处理
observations.push_back(obs);
}
file.close();
return observations;
}
int main() {
try {
const std::string rinex_file = "path_to_your_rinex_file.rnx";
auto observations = readRinexFile(rinex_file);
// 现在你可以遍历并处理 observations 数组
} catch (const std::exception& e) {
std::cerr << "错误:" << e.what() << std::endl;
}
return 0;
}
```
请注意,这只是一个基础示例,实际的RINEX文件格式非常复杂,包括头文件、站名标识符、观测数据等多个部分,解析起来可能需要专门的库或自定义更复杂的解析逻辑。
阅读全文
相关推荐



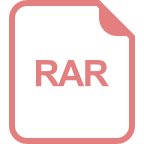











