python混淆矩阵画图颜色
时间: 2023-08-28 16:22:33 浏览: 270
在Python中,你可以使用`matplotlib`库来画混淆矩阵,并使用不同的颜色来表示不同的类别。下面是一个简单的例子:
```python
import numpy as np
import matplotlib.pyplot as plt
# 创建一个混淆矩阵
confusion_matrix = np.array([[10, 2, 3],
[4, 15, 6],
[7, 8, 20]])
# 设置类别标签
labels = ['Class 1', 'Class 2', 'Class 3']
# 设置颜色映射
colors = ['blue', 'green', 'red']
# 绘制混淆矩阵图
plt.figure(figsize=(8, 6))
plt.imshow(confusion_matrix, cmap='Blues') # 使用蓝色作为背景色
# 添加颜色条
cbar = plt.colorbar()
cbar.set_label('Count')
# 添加类别标签
阅读全文
相关推荐
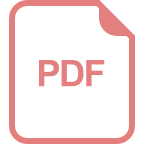
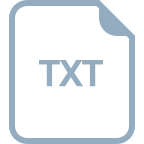
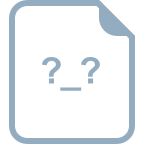
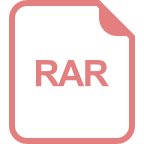
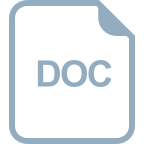
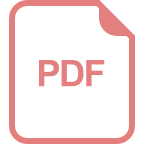
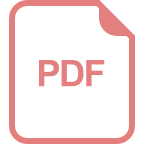
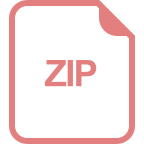
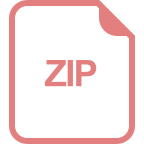
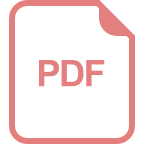
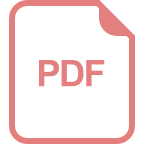