如何在C++中编写计算三点连成两条直线之间夹角的代码
时间: 2024-10-18 18:11:19 浏览: 24
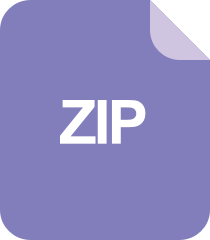
两条直线(四点坐标)计算直线夹角

在C++中,我们可以使用向量运算和三角函数来计算两点之间的线夹角。首先,我们需要确定三个点A、B、C,其中A和B确定了线段的方向,而C在线段上或其延长线上。线段AB和AC的向量分别是v1 = B - A 和 v2 = C - A。下面是一个简单的示例:
```cpp
#include <cmath>
#include <iostream>
// 向量类
class Vector {
public:
double x, y;
// 构造函数
Vector(double x0, double y0) : x(x0), y(y0) {}
// 向量减法
Vector operator-(const Vector& other) const {
return {x - other.x, y - other.y};
}
// 向量长度
double magnitude() const {
return std::sqrt(x * x + y * y);
}
// 向量内积(点积)
double dot(const Vector& other) const {
return x * other.x + y * other.y;
}
// 向量叉积(外积)
double cross(const Vector& other) const {
return x * other.y - y * other.x;
}
};
// 计算两个向量之间的角度 (弧度制)
double angleBetweenVectors(const Vector& v1, const Vector& v2) {
double dotProduct = v1.dot(v2);
double magnitudeProduct = v1.magnitude() * v2.magnitude();
if (magnitudeProduct == 0) {
return std::atan2(0, 0); // 防止除零错误,这里通常返回π/2或-π/2
} else {
return std::acos(dotProduct / magnitudeProduct);
}
}
int main() {
// 定义三点坐标
Vector A(0, 0), B(1, 0), C(0.5, 0.866); // 假设B在A右边,C在AB线上
// 计算线段AB和AC之间的夹角
double angleABC = angleBetweenVectors(B - A, C - A);
// 将角度转换为角度制(如果需要)
std::cout << "The angle between AB and AC is: " << std::to_string(angleABC * 180 / M_PI) << " degrees.\n";
return 0;
}
```
阅读全文
相关推荐
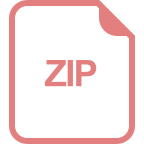
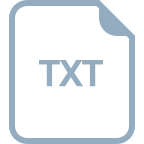
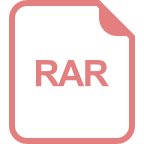
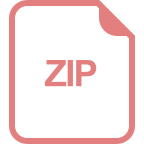
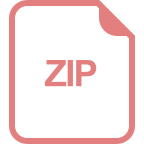
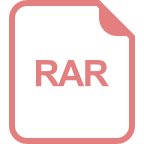
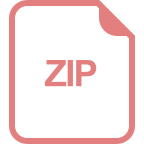
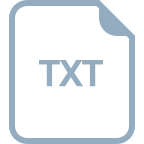
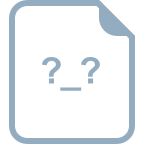
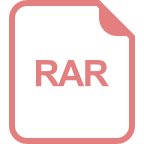
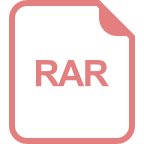
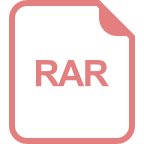
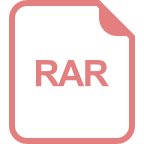
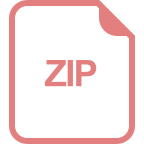
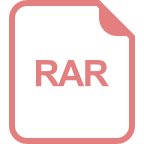
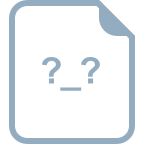