python图像文件压缩7.2
时间: 2024-05-19 16:09:44 浏览: 125
Python 中可以使用 Pillow 库来处理图像文件压缩。Pillow 是 Python 中一个非常流行的图像处理库,支持各种图像格式的读取和处理,包括但不限于 JPEG、PNG、GIF 等等。以下是一个基于 Pillow 库的图像文件压缩的示例代码:
```python
from PIL import Image
def compress_image(infile, outfile):
try:
with Image.open(infile) as im:
im.save(outfile, optimize=True, quality=72)
except OSError:
print("cannot compress", infile)
# 使用示例
compress_image("input.jpg", "output.jpg")
```
以上代码中,`compress_image` 函数接受两个参数,分别是输入文件路径和输出文件路径。在函数中,我们使用 `with` 语句打开输入文件,并使用 `save` 方法将其保存为输出文件。`optimize=True` 参数表示启用优化,`quality=72` 参数表示设定压缩质量为 72。
如果要对多个文件进行压缩,可以使用 `os` 模块遍历目录,并对每个图像文件调用 `compress_image` 函数。例如:
```python
import os
def compress_images_in_folder(folder):
for dirpath, dirnames, filenames in os.walk(folder):
for filename in filenames:
if filename.lower().endswith(".jpg") or filename.lower().endswith(".jpeg"):
infile = os.path.join(dirpath, filename)
outfile = os.path.join(dirpath, "compressed_" + filename)
compress_image(infile, outfile)
# 使用示例
compress_images_in_folder("/path/to/folder")
```
以上代码中,`compress_images_in_folder` 函数接受一个参数,即要进行压缩的目录路径。函数使用 `os.walk` 遍历目录下的所有文件和子目录,并对每个后缀名为 `.jpg` 或者 `.jpeg` 的文件调用 `compress_image` 函数,将压缩后的文件保存在原目录下,并添加前缀 "compressed_"。
阅读全文
相关推荐
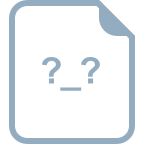
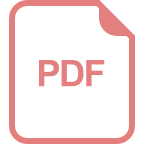

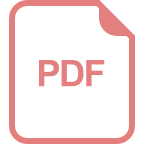
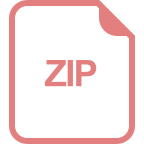
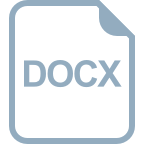
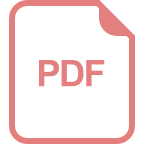
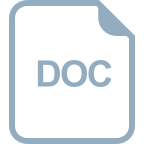
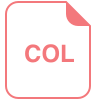
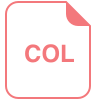
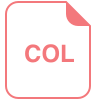
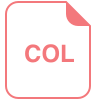
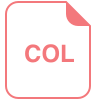
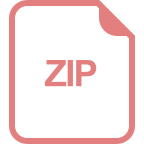
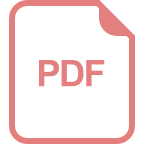