轨道距离函数用python计算怎么写代码
时间: 2024-04-11 20:02:29 浏览: 11
轨道距离(Hausdorff距离)是用来度量两个轨迹或线段之间的相似性或距离的一种方法。下面是使用Python计算轨道距离的示例代码:
```python
import numpy as np
from scipy.spatial.distance import directed_hausdorff
# 假设有两个轨迹,每个轨迹由一系列点表示
trajectory1 = np.array([[0, 0], [1, 1], [2, 2]])
trajectory2 = np.array([[2, 2], [3, 3], [4, 4]])
# 计算轨道距离
distance, _ = directed_hausdorff(trajectory1, trajectory2)
print("轨道距离:", distance)
```
在这个示例中,`trajectory1`和`trajectory2`分别表示两个轨迹,每个轨迹由一系列点的坐标组成。使用`directed_hausdorff`函数从scipy库计算轨道距离。函数返回两个轨迹之间的距离以及在该距离下的最佳配对。
请注意,输入的轨迹必须是具有相同维度的NumPy数组,其中每个点的坐标表示为一个数组。此外,如果您的轨迹是时间序列数据,则可能需要进行预处理以确保数据点的时间先后顺序正确。
这只是一个简单的示例,您可以根据实际需求进行适当的调整和扩展。
相关问题
Python写街区距离的计算函数
可以使用以下代码实现街区距离的计算函数:
```python
def manhattan_distance(x1, y1, x2, y2):
"""
计算两点之间的街区距离
:param x1: 第一个点的横坐标
:param y1: 第一个点的纵坐标
:param x2: 第二个点的横坐标
:param y2: 第二个点的纵坐标
:return: 两点之间的街区距离
"""
return abs(x1 - x2) + abs(y1 - y2)
```
使用方法:
```python
distance = manhattan_distance(1, 2, 4, 6)
print(distance) # 输出结果为 7
```
其中,`x1`、`y1`表示第一个点的横纵坐标,`x2`、`y2`表示第二个点的横纵坐标。函数返回值为两点之间的街区距离。
python写一个计算交叉熵损失函数的代码
当使用Python编写计算交叉熵损失函数的代码时,可以使用以下代码示例:
```python
import numpy as np
def cross_entropy_loss(y_true, y_pred):
epsilon = 1e-7 # 避免log(0)的情况
y_pred = np.clip(y_pred, epsilon, 1.0 - epsilon) # 将预测值限制在一个较小的范围内,避免出现无穷大的情况
loss = -np.sum(y_true * np.log(y_pred)) # 计算交叉熵损失
return loss
# 示例用法
y_true = np.array([0, 1, 0]) # 真实标签
y_pred = np.array([0.2, 0.7, 0.1]) # 预测概率
loss = cross_entropy_loss(y_true, y_pred)
print("交叉熵损失:", loss)
```
上述代码中,`cross_entropy_loss`函数接受两个参数:`y_true`表示真实标签,`y_pred`表示预测概率。函数首先对预测概率进行了限制,避免出现无穷大的情况。然后,使用numpy库中的函数计算交叉熵损失。最后,返回计算得到的损失值。
相关推荐
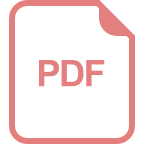












