base64解码 C语言实现 openssl
时间: 2023-07-21 19:10:54 浏览: 210
在 C 语言中,您可以使用 OpenSSL 库来实现 Base64 解码。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bio.h>
#include <openssl/evp.h>
int base64_decode(const unsigned char* encoded_data, size_t encoded_length, unsigned char* decoded_data, size_t* decoded_length) {
BIO *bio, *b64;
size_t length = 0;
// 创建 Base64 解码的 BIO
b64 = BIO_new(BIO_f_base64());
bio = BIO_new_mem_buf(encoded_data, encoded_length);
bio = BIO_push(b64, bio);
// 解码
BIO_set_flags(bio, BIO_FLAGS_BASE64_NO_NL);
length = BIO_read(bio, decoded_data, encoded_length);
// 清理并设置解码后的长度
BIO_free_all(bio);
*decoded_length = length;
return 0;
}
int main() {
const char* encoded_text = "SGVsbG8gd29ybGQh"; // 输入要解码的 Base64 编码字符串
size_t encoded_length = strlen(encoded_text);
size_t decoded_length = ((encoded_length + 3) / 4) * 3;
unsigned char* decoded_text = (unsigned char*)malloc(decoded_length + 1);
if (decoded_text == NULL) {
printf("内存分配失败!\n");
return 1;
}
int result = base64_decode((const unsigned char*)encoded_text, encoded_length, decoded_text, &decoded_length);
if (result != 0) {
printf("解码失败:%d\n", result);
free(decoded_text);
return 1;
}
decoded_text[decoded_length] = '\0';
printf("解码结果:%s\n", decoded_text);
free(decoded_text);
return 0;
}
```
在上述示例代码中,我们使用了 OpenSSL 的 `BIO`(I/O 抽象)和 `EVP`(加密算法)来实现 Base64 解码。需要注意的是,您需要先安装 OpenSSL 库并确保编译时链接了正确的库文件。
编译和运行上述代码后,将输出解码结果:"Hello world!"。
请注意,此示例仅演示了如何使用 OpenSSL 库来进行 Base64 解码。如果您需要进行更复杂的加密/解密操作,可以参考 OpenSSL 的官方文档或其他资源获取更多信息。
阅读全文
相关推荐
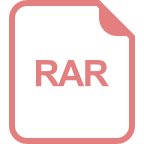

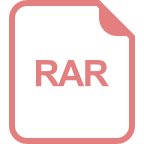





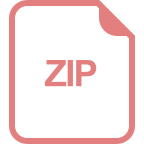
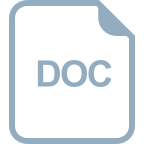





