用c语言编写一个程序,定义并调用一个函数,该函数接收一个整数数组,返回数组中的最大值及其索引
时间: 2024-11-05 19:28:53 浏览: 6
在 C 语言中,你可以定义一个函数 `findMax` 来找到数组中的最大值及其索引。这里是一个简单的示例:
```c
#include <stdio.h>
#include <limits.h> // 包含 INT_MAX 用于设置初始的最大值
// 定义函数 findMax,接收一个整数数组和其长度作为参数
void findMax(int arr[], int n, int* maxVal, int* maxIndex) {
*maxVal = INT_MIN; // 初始化最大值为负无穷
*maxIndex = -1; // 初始化最大值索引为 -1
for (int i = 0; i < n; i++) { // 遍历数组
if (arr[i] > *maxVal) { // 如果当前元素大于已知的最大值
*maxVal = arr[i]; // 更新最大值
*maxIndex = i; // 更新最大值的索引
}
}
}
int main() {
int numbers[] = {5, 9, 1, 7, 3};
int arrayLength = sizeof(numbers) / sizeof(numbers[0]);
int maxValue, maxIndex;
findMax(numbers, arrayLength, &maxValue, &maxIndex); // 调用函数并传入地址以修改变量值
printf("The maximum value is %d at index %d\n", maxValue, maxIndex);
return 0;
}
```
在这个程序中,我们首先定义了一个名为 `findMax` 的函数,它接受一个整数数组、数组长度以及指向最大值和索引的指针。函数内部遍历整个数组,如果发现新的最大值,就更新最大值和对应的索引。
在 `main` 函数里,我们创建了一个整数数组,然后调用 `findMax` 函数并将结果保存在 `maxValue` 和 `maxIndex` 变量中。最后打印出最大值和它的索引。
阅读全文
相关推荐
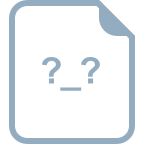
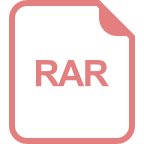















