嵌入式liunx线程编程c语言代码
时间: 2024-08-12 09:07:00 浏览: 38
嵌入式Linux下的线程编程通常使用POSIX线程库(pthread)在C语言中进行。以下是一个简单的示例,展示了如何创建、启动和同步线程:
```c
#include <stdio.h>
#include <pthread.h>
// 线程执行的函数
void* thread_function(void* arg) {
int my_id = (int)arg;
printf("Thread %d started\n", my_id);
// 这里是线程的具体任务,例如处理数据或执行某个循环
for (int i = 0; i < 5; i++) {
printf("Thread %d: Loop %d\n", my_id, i);
sleep(1); // 暂停1秒模拟任务执行
}
printf("Thread %d finished\n", my_id);
return NULL;
}
int main() {
pthread_t thread1, thread2; // 创建线程的句柄
// 创建并启动线程1
int thread1_id = 1;
if (pthread_create(&thread1, NULL, thread_function, (void*)&thread1_id) != 0) {
perror("Error creating thread 1");
return 1;
}
// 创建并启动线程2
int thread2_id = 2;
if (pthread_create(&thread2, NULL, thread_function, (void*)&thread2_id) != 0) {
perror("Error creating thread 2");
return 1;
}
// 等待线程完成
pthread_join(thread1, NULL); // 线程1等待
pthread_join(thread2, NULL); // 线程2等待
printf("Main thread finished\n");
return 0;
}
```
在这个例子中:
- `pthread_create`函数用于创建新线程,它接收线程标识符、线程属性指针、线程函数指针和参数指针。
- `thread_function`是每个线程实际执行的任务。
- `pthread_join`用于让主线程等待子线程完成。
相关推荐
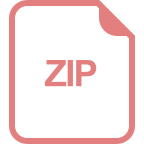
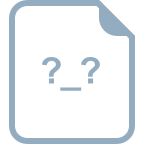
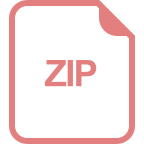














