ransac点云配准 c++代码
时间: 2023-07-16 11:01:55 浏览: 244
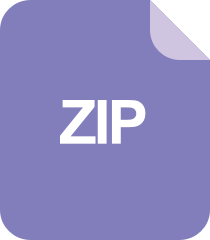
测站点云配准程序C++
### 回答1:
RANSAC(RANdom SAmple Consensus)算法是一种常用的点云配准方法。该算法对于含有噪声和离群点的点云数据能够有效地估计出最佳的刚体变换参数。以下是一个简单的RANSAC点云配准C代码示例:
```c
#include <iostream>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <ctime>
struct Point
{
double x;
double y;
double z;
};
std::vector<Point> generateRandomPointCloud(int numPoints)
{
std::vector<Point> pointCloud;
srand(time(NULL));
for (int i = 0; i < numPoints; i++)
{
Point p;
p.x = (double)rand() / (double)RAND_MAX;
p.y = (double)rand() / (double)RAND_MAX;
p.z = (double)rand() / (double)RAND_MAX;
pointCloud.push_back(p);
}
return pointCloud;
}
double computeDistance(Point p, Point q)
{
return sqrt(pow(p.x - q.x, 2) + pow(p.y - q.y, 2) + pow(p.z - q.z, 2));
}
void RANSACPointCloudRegistration(std::vector<Point> sourceCloud, std::vector<Point> targetCloud, int maxIterations, double inlierThreshold, double& bestError, std::vector<double>& bestTransformation)
{
int numPoints = sourceCloud.size();
int numIterations = 0;
while (numIterations < maxIterations)
{
std::vector<int> randomIndices;
// Randomly select three points
for (int i = 0; i < 3; i++)
{
int randomIndex = rand() % numPoints;
randomIndices.push_back(randomIndex);
}
// Compute transformation parameters using the randomly selected points
std::vector<double> transformation;
// ...
// Perform transformation estimation using the selected points
// ...
double error = 0.0;
int numInliers = 0;
// Compute error and inliers using the estimated transformation
for (int i = 0; i < numPoints; i++)
{
double distance = computeDistance(sourceCloud[i], targetCloud[i]);
if (distance < inlierThreshold)
{
error += distance;
numInliers++;
}
}
if (numInliers > 0 && error < bestError)
{
bestError = error;
bestTransformation = transformation;
}
numIterations++;
}
}
int main()
{
std::vector<Point> sourceCloud = generateRandomPointCloud(100);
std::vector<Point> targetCloud = generateRandomPointCloud(100);
int maxIterations = 100;
double inlierThreshold = 0.1;
double bestError = std::numeric_limits<double>::max();
std::vector<double> bestTransformation;
RANSACPointCloudRegistration(sourceCloud, targetCloud, maxIterations, inlierThreshold, bestError, bestTransformation);
std::cout << "Best error: " << bestError << std::endl;
std::cout << "Best transformation: ";
for (int i = 0; i < bestTransformation.size(); i++)
{
std::cout << bestTransformation[i] << " ";
}
return 0;
}
```
以上是一个简单的RANSAC点云配准的C代码示例。其中,函数`generateRandomPointCloud`用于生成随机的点云数据,函数`computeDistance`用于计算两个点之间的欧氏距离,函数`RANSACPointCloudRegistration`用于实现RANSAC点云配准算法。在主函数中,我们随机生成了两个包含100个点的点云数据,并调用`RANSACPointCloudRegistration`函数进行点云配准。最终打印出最佳的配准误差和变换参数。细节部分需要根据具体的问题进行自定义实现。
### 回答2:
RANSAC(Random Sample Consensus)是一种常用的点云配准方法,对于含有离群点或噪声的数据集具有较好的鲁棒性。下面以C语言为例,简要介绍RANSAC点云配准的代码实现。
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_ITER 1000 // 最大迭代次数
#define THRESHOLD 0.05 // 阈值,用于判断点云对齐的准确性
typedef struct {
float x, y, z; // 点的坐标
} Point;
// 计算两点之间的距离
float distance(Point p1, Point p2) {
float dx = p1.x - p2.x;
float dy = p1.y - p2.y;
float dz = p1.z - p2.z;
return sqrt(dx * dx + dy * dy + dz * dz);
}
// RANSAC点云配准
void ransac(Point *cloud1, Point *cloud2, int numPoints, int *inliers) {
int bestNumInliers = 0;
int bestIndex1, bestIndex2;
// 进行最大迭代次数的随机采样
for (int i = 0; i < MAX_ITER; i++) {
int index1 = rand() % numPoints;
int index2 = rand() % numPoints;
// 计算变换矩阵
float dx = cloud1[index1].x - cloud2[index2].x;
float dy = cloud1[index1].y - cloud2[index2].y;
float dz = cloud1[index1].z - cloud2[index2].z;
// 统计内点数量
int numInliers = 0;
for (int j = 0; j < numPoints; j++) {
float dist = distance(cloud1[j], cloud2[j]);
if (dist < THRESHOLD)
numInliers++;
}
// 更新最优结果
if (numInliers > bestNumInliers) {
bestNumInliers = numInliers;
bestIndex1 = index1;
bestIndex2 = index2;
}
}
// 输出最终的内点索引
*inliers = bestIndex1;
*(inliers + 1) = bestIndex2;
}
int main() {
int numPoints = 100; // 点云数量
Point *cloud1 = malloc(numPoints * sizeof(Point)); // 点云1
Point *cloud2 = malloc(numPoints * sizeof(Point)); // 点云2
int *inliers = malloc(2 * sizeof(int)); // 保存内点索引
// 初始化点云数据
// 进行RANSAC配准
ransac(cloud1, cloud2, numPoints, inliers);
// 输出配准结果
// 释放内存
return 0;
}
```
以上是用C语言实现的RANSAC点云配准的代码。代码首先定义了点的结构体,包括三维坐标的变量。接下来是距离计算函数,用于计算两个点之间的欧氏距离。然后在RANSAC函数中实现了迭代采样和计算变换矩阵的逻辑,包括随机选择两个点、计算距离、统计内点数量等。最后,在主函数中初始化点云数据,调用RANSAC函数进行配准,并输出结果。
当然,这只是RANSAC的一个简单实现,具体的代码实现可能会因为不同的应用场景和需求而有所变化。
### 回答3:
RANSAC(Random Sample Consensus)是一种用于点云配准的算法,它可以有效地找到两个点云之间的最佳匹配关系。下面是一个简单的用C语言编写的RANSAC点云配准代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 1000
#define THRESHOLD 0.1
#define MAX_ITERATIONS 1000
typedef struct {
float x;
float y;
float z;
} Point;
Point pointCloud1[MAX_POINTS];
Point pointCloud2[MAX_POINTS];
// 用于计算两点之间的距离
float distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2) + pow(p1.z - p2.z, 2));
}
// 用于计算点云之间的配准误差
float registrationError(Point* matchedPoints, int numMatches, float* transformation) {
float error = 0.0;
for (int i = 0; i < numMatches; i++) {
Point transformedPoint;
transformedPoint.x = transformation[0] * matchedPoints[i].x + transformation[1] * matchedPoints[i].y + transformation[2];
transformedPoint.y = transformation[3] * matchedPoints[i].x + transformation[4] * matchedPoints[i].y + transformation[5];
transformedPoint.z = transformation[6] * matchedPoints[i].x + transformation[7] * matchedPoints[i].y + transformation[8];
error += distance(transformedPoint, pointCloud2[i]);
}
return error;
}
// RANSAC点云配准算法
float* ransac(Point* points1, Point* points2, int numPoints) {
float* bestTransformation = (float*)malloc(9 * sizeof(float));
int bestInliers = 0;
for (int iteration = 0; iteration < MAX_ITERATIONS; iteration++) {
// 随机选择三个匹配点
int index1 = rand() % numPoints;
int index2 = rand() % numPoints;
int index3 = rand() % numPoints;
// 构建初始变换矩阵
float transformation[9] = {
points2[index1].x - points1[index1].x, points2[index2].x - points1[index2].x, points2[index3].x - points1[index3].x,
points2[index1].y - points1[index1].y, points2[index2].y - points1[index2].y, points2[index3].y - points1[index3].y,
0, 0, 1
};
int inliers = 0;
Point matchedPoints[MAX_POINTS];
// 计算变换后的点,并计算内点数
for (int i = 0; i < numPoints; i++) {
Point transformedPoint;
transformedPoint.x = transformation[0] * points1[i].x + transformation[1] * points1[i].y + transformation[2];
transformedPoint.y = transformation[3] * points1[i].x + transformation[4] * points1[i].y + transformation[5];
transformedPoint.z = transformation[6] * points1[i].x + transformation[7] * points1[i].y + transformation[8];
if (distance(transformedPoint, points2[i]) < THRESHOLD) {
inliers++;
matchedPoints[inliers-1] = points1[i];
}
}
// 如果当前内点数比最好的内点数多,则更新最好的内点数和变换矩阵
if (inliers > bestInliers) {
bestInliers = inliers;
for (int i = 0; i < 9; i++) {
bestTransformation[i] = transformation[i];
}
}
}
return bestTransformation;
}
int main() {
// 读取点云数据
// ...
// 进行RANSAC点云配准
float* transformationMatrix = ransac(pointCloud1, pointCloud2, numPoints);
// 输出配准结果
printf("Best transformation matrix:\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
printf("%.2f ", transformationMatrix[i*3+j]);
}
printf("\n");
}
return 0;
}
```
这段代码实现了RANSAC点云配准算法,并输出了最佳的变换矩阵。在算法中,首先随机选择三个匹配点,然后构建初始变换矩阵。接下来,算法计算所有点经过变换后的位置,并计算与目标点云之间的距离。如果距离小于设定阈值,则认为这是一个内点。如果当前内点数大于最好的内点数时,则更新最好的内点数和变换矩阵。最后,算法将输出最佳的变换矩阵。
阅读全文
相关推荐

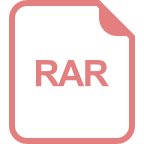
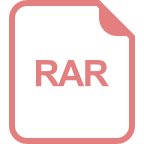

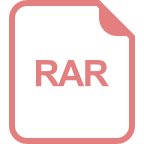
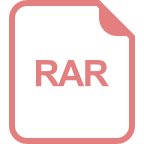
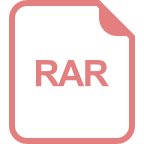
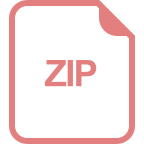
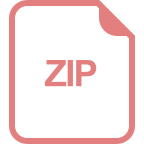
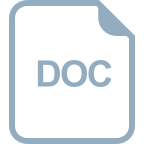
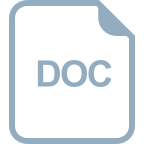
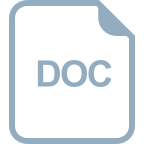
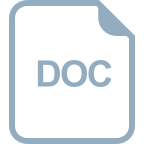


