请使用常用的运算符编写一个java程序。要求用到算术运算符、关系运算符、逻辑运算符、位运算符等
时间: 2024-09-30 10:12:30 浏览: 39
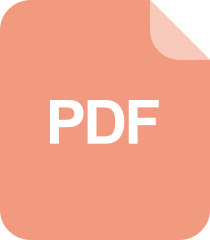
day02-运算符及控制台.pdf
下面是一个简单的Java程序,展示了各种运算符的用法:
```java
public class OperationExample {
public static void main(String[] args) {
// 算术运算符
int a = 10;
int b = 5;
int sum = a + b; // 加法
int difference = a - b; // 减法
int product = a * b; // 乘法
int quotient = a / b; // 整除
double remainder = a % b; // 取余数
// 关系运算符
boolean isGreater = a > b;
boolean isLess = a < b;
boolean isEqual = a == b;
// 逻辑运算符
boolean bothTrue = a > 0 && b > 0; // 与操作
boolean eitherTrue = a > 0 || b > 0; // 或操作
boolean notTrue = !a > 0; // 非操作
// 位运算符
int bitwiseAnd = a & b; // 按位与
int bitwiseOr = a | b; // 按位或
int bitwiseXor = a ^ b; // 按位异或
int bitwiseNotA = ~a; // 按位取反
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
System.out.println("Remainder: " + remainder);
System.out.println("Is a greater than b? " + isGreater);
System.out.println("Is a less than b? " + isLess);
System.out.println("Are they equal? " + isEqual);
System.out.println("Both true? " + bothTrue);
System.out.println("Either true? " + eitherTrue);
System.out.println("Not true? " + notTrue);
System.out.println("Bitwise and: " + bitwiseAnd);
System.out.println("Bitwise or: " + bitwiseOr);
System.out.println("Bitwise xor: " + bitwiseXor);
System.out.println("Bitwise not of a: " + bitwiseNotA);
}
}
```
阅读全文
相关推荐
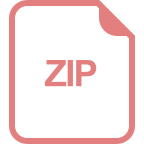
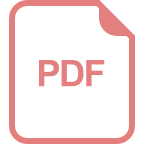

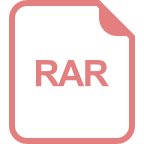
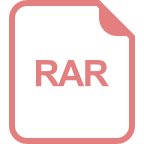
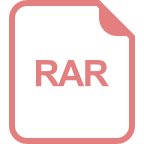
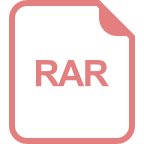
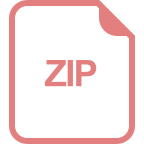
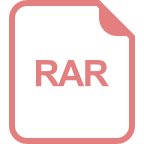
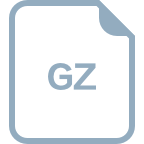
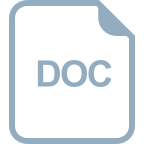
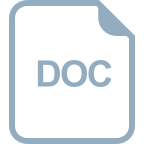
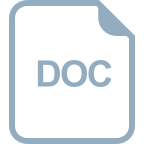
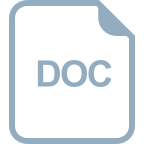
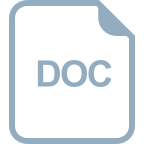
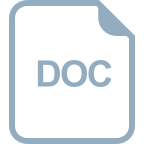
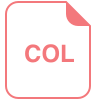
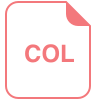