栅格地图AGV路径规划python
时间: 2024-09-10 21:23:00 浏览: 40
栅格地图是一种将环境划分为规则格子的形式来表示的二维地图。在这种地图中,每个格子可以被标记为可通行或不可通行,用以表示障碍物和其他特征。AGV(自动引导车)路径规划是指在这样的栅格地图上找到从起点到终点的一条有效路径,同时要尽量避免碰撞障碍物,并可能需要满足其他诸如最短距离、最少转弯次数等优化条件。
在Python中进行AGV路径规划,我们通常会采用一些算法来实现,比如A*算法、Dijkstra算法或者深度优先搜索(DFS)算法等。以A*算法为例,这是一种启发式搜索算法,它结合了最好优先搜索和Dijkstra算法的优点。A*算法使用一个估价函数f(n) = g(n) + h(n),其中g(n)是从起点到当前点的实际代价,而h(n)是从当前点到目标点的估计代价。h(n)通常是启发式的,例如使用欧几里得距离或者曼哈顿距离作为估计。
下面是一个简单的A*算法实现栅格地图AGV路径规划的Python代码示例(注意:这个示例仅供参考,实际应用中需要根据具体环境进行调整):
```python
import heapq
class Node:
def __init__(self, parent=None, position=None):
self.parent = parent
self.position = position
self.g = 0
self.h = 0
self.f = 0
def __eq__(self, other):
return self.position == other.position
def __lt__(self, other):
return self.f < other.f
def astar(maze, start, end):
start_node = Node(None, tuple(start))
end_node = Node(None, tuple(end))
open_list = []
closed_list = set()
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node)
if current_node == end_node:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1] # Return reversed path
children = []
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]: # Adjacent squares
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if node_position[0] > (len(maze) - 1) or node_position[0] < 0 or node_position[1] > (len(maze[len(maze)-1]) -1) or node_position[1] < 0:
continue
if maze[node_position[0]][node_position[1]] != 0:
continue
new_node = Node(current_node, node_position)
children.append(new_node)
for child in children:
if child in closed_list:
continue
child.g = current_node.g + 1
child.h = ((child.position[0] - end_node.position[0]) ** 2) + ((child.position[1] - end_node.position[1]) ** 2)
child.f = child.g + child.h
if len([open_node for open_node in open_list if child == open_node and child.g > open_node.g]) > 0:
continue
heapq.heappush(open_list, child)
return None
# Example usage:
maze = [[0, 0, 0, 0, 1],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]]
start = [0, 0]
end = [4, 4]
path = astar(maze, start, end)
print(path)
```
阅读全文
相关推荐
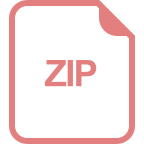
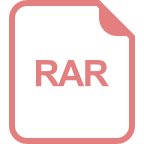
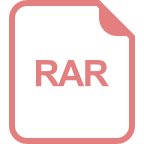















