51单片机利用定时器和数码管,完成按键按下几秒,数码管显示几(向下取整)
时间: 2024-11-09 09:29:41 浏览: 16
要使用51单片机的定时器配合数码管实现这个功能,你需要进行以下几个步骤:
1. **初始化硬件**:
- 首先,确保连接了正确的数码管接口和按键,通常数码管使用7段LED或共阳极/共阴极接法。
- 初始化定时器,比如使用T0或T1,设置为模式1的定时器中断,以便于计数。
2. **设置定时器的工作模式**:
- 对于定时器T0,你可以使用MOV TH0, #0xXX 和 MOV TL0, #0xYY 来设置高8位和低8位计数初值,其中XX和YY是你要设定的初始时间间隔。
3. **启用定时器中断**:
- 打开定时器的中断源,例如 SETB ET0 (对于T0) 或 SETB EX0 (对于T1),并设置相应的中断标志位如IE0 (T0) 或 TF0 (T1)。
4. **处理中断**:
- 编写ISR(Interrupt Service Routine),当定时器溢出时被触发。在这个函数里,你可以减去设定的时间间隔,然后检查是否还有剩余时间。
5. **控制数码管显示**:
- 当剩余时间为0或小于1秒时,根据按键的状态更新数码管显示。如果按键持续按下,则减小显示数值;如果按键松开则显示下一位数字。
6. **向下取整**:
- 使用除法或者位操作技巧,保证每次显示的数字都是整数。例如,你可以用定时器的计数值除以10(或更高的基数),然后将余数丢弃,得到下一个整数。
下面是简化版的伪代码示例:
```c
#include <reg51.h> // Include the appropriate header file for your specific microcontroller
// Define constants and variables
const int TIMER_INTERVAL = 1000; // Set desired interval in milliseconds
int display_value = 0;
bool key_pressed = false;
void timer_isr() interrupt 1 {
if (key_pressed) {
display_value++;
if (display_value >= 10) { // Assuming a 4-digit display
display_value = 0;
}
}
// Update the timer value (subtracting the interval)
// ... (your code here to update TL0 and TH0)
// Clear the interrupt flag
-clear TF0;
}
void main() {
// Initialize hardware, set up timers and interrupts
// ...
// Enable interrupts
SETB EA;
while(1) {
// Check for key press and update state
// ... (your code here to read and store key state)
// If key is pressed, start the timer
if (key_pressed) {
clear_timer();
start_timer(TIMER_INTERVAL);
} else {
stop_timer(); // Stop or pause the timer
}
}
}
```
记得替换`clear_timer()`、`start_timer()`和`stop_timer()`这些函数的实际实现,以及根据你的具体硬件调整代码。
阅读全文
相关推荐
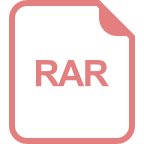
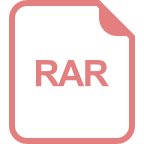
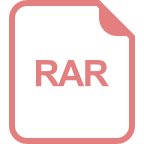

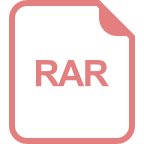
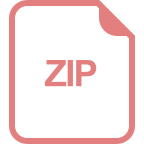
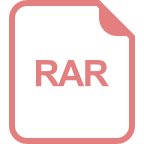
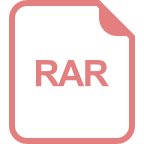
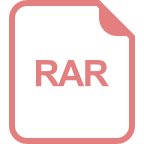
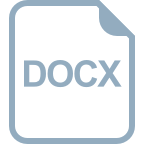
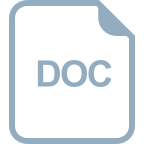
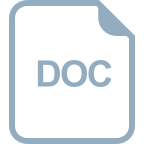
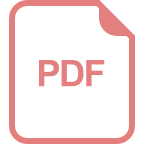
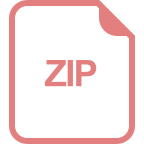
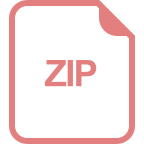
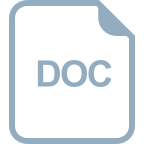
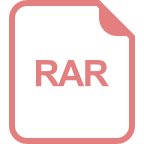
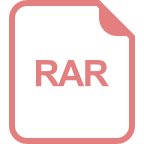
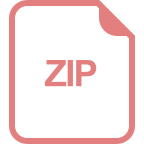