C++语言实现开心消消乐
时间: 2023-10-07 12:11:32 浏览: 67
开心消消乐是一款简单的益智游戏,玩家需要通过消除相邻的相同图案来得分。以下是一个使用C++实现的基本游戏框架:
```cpp
#include<iostream>
#include<conio.h>
#include<cstdlib>
#include<ctime>
using namespace std;
const int ROW = 8;
const int COL = 8;
const int COLORS = 4;
int score = 0;
int board[ROW][COL];
void printBoard();
void initBoard();
bool checkEmpty();
void swap(int&, int&);
bool checkMatch(int, int);
void removeMatches();
void fillEmpty();
int main(){
srand(time(NULL));
initBoard();
printBoard();
while(checkEmpty()==false){
int x1,y1,x2,y2;
cout<<"Enter the row and column of the first tile to swap: ";
cin>>x1>>y1;
cout<<"Enter the row and column of the second tile to swap: ";
cin>>x2>>y2;
swap(board[x1][y1],board[x2][y2]);
if(checkMatch(x1,y1) || checkMatch(x2,y2)){
removeMatches();
fillEmpty();
cout<<"Score: "<<score<<endl;
printBoard();
}
else{
swap(board[x1][y1],board[x2][y2]);
cout<<"Invalid move"<<endl;
}
}
cout<<"Game Over!"<<endl;
return 0;
}
void printBoard(){
cout<<" ";
for(int i=0;i<COL;i++){
cout<<i<<" ";
}
cout<<endl;
for(int i=0;i<ROW;i++){
cout<<i<<" ";
for(int j=0;j<COL;j++){
cout<<board[i][j]<<" ";
}
cout<<endl;
}
}
void initBoard(){
for(int i=0;i<ROW;i++){
for(int j=0;j<COL;j++){
board[i][j] = rand()%COLORS+1;
}
}
}
bool checkEmpty(){
for(int i=0;i<ROW;i++){
for(int j=0;j<COL;j++){
if(board[i][j]!=0){
return false;
}
}
}
return true;
}
void swap(int& a, int& b){
int temp = a;
a = b;
b = temp;
}
bool checkMatch(int x, int y){
int color = board[x][y];
int count = 1;
int i = x-1;
while(i>=0 && board[i][y]==color){
count++;
i--;
}
i = x+1;
while(i<ROW && board[i][y]==color){
count++;
i++;
}
if(count>=3){
return true;
}
count = 1;
int j = y-1;
while(j>=0 && board[x][j]==color){
count++;
j--;
}
j = y+1;
while(j<COL && board[x][j]==color){
count++;
j++;
}
if(count>=3){
return true;
}
return false;
}
void removeMatches(){
for(int i=0;i<ROW;i++){
for(int j=0;j<COL;j++){
if(board[i][j]!=0 && checkMatch(i,j)){
board[i][j] = 0;
score++;
}
}
}
}
void fillEmpty(){
for(int j=0;j<COL;j++){
for(int i=ROW-1;i>=0;i--){
if(board[i][j]==0){
int k = i-1;
while(k>=0 && board[k][j]==0){
k--;
}
if(k>=0){
swap(board[i][j],board[k][j]);
}
else{
board[i][j] = rand()%COLORS+1;
}
}
}
}
}
```
这是一个基本的实现,你可以根据需要进行修改和改进。
相关推荐
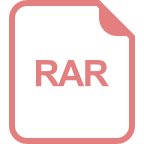
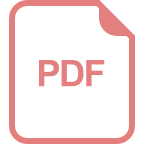














