怎么给iframe传值
时间: 2023-08-17 09:10:14 浏览: 209
你可以通过以下几种方法给一个iframe传值:
1. 使用URL参数:你可以在iframe的URL中添加参数来传递值。在父页面中,使用`src`属性来指定iframe的URL,并在URL末尾添加参数,例如:`<iframe src="child.html?value=123"></iframe>`。在子页面中,你可以使用JavaScript来获取URL参数并处理它。
2. 使用postMessage方法:postMessage是HTML5中提供的一种跨窗口通信的方法。通过postMessage方法,你可以在父页面和子页面之间传递数据。在父页面中,你可以使用`contentWindow.postMessage()`方法来向子页面发送消息。在子页面中,你可以通过监听`message`事件来接收并处理这些消息。
3. 使用JavaScript全局对象:如果你在父页面和子页面之间共享相同的全局对象(例如window对象),你可以直接在父页面中设置全局对象的属性,并在子页面中访问该属性来获取传递的值。
以上是几种常见的给iframe传值的方法,你可以根据具体的需求选择合适的方法来实现。
相关问题
vue给iframe传值
可以使用postMessage方法来实现vue给iframe传值。具体步骤如下:
1. 在vue中,获取到需要传递的数据。
2. 获取到iframe元素,通过contentWindow属性获取到iframe的window对象。
3. 调用window对象的postMessage方法,将数据发送到iframe中。
示例代码如下:
```javascript
// 获取到需要传递的数据
const data = { name: '张三', age: 20 };
// 获取到iframe元素
const iframe = document.getElementById('my-iframe');
// 获取到iframe的window对象
const iframeWindow = iframe.contentWindow;
// 将数据发送到iframe中
iframeWindow.postMessage(data, '*');
```
在iframe中,可以通过监听message事件来获取到vue传递过来的数据。示例代码如下:
```javascript
// 监听message事件
window.addEventListener('message', function(event) {
// 获取到传递过来的数据
const data = event.data;
console.log(data);
});
```
需要注意的是,由于postMessage方法可以跨域传递数据,因此需要在接收数据的iframe中对数据来源进行验证,以确保数据安全。
vue3+ts给iframe传值
在 Vue 3 中,你可以使用 `ref` 来创建一个对 DOM 元素的引用,并使用 `$refs` 来访问它。在 TypeScript 中,你需要使用 `Ref` 类型来定义引用。
以下是一个示例代码,展示如何在 Vue 3 + TypeScript 中使用 `ref` 与 `postMessage` 传递数据给 `iframe`:
```vue
<template>
<iframe ref="myIframe" src="https://example.com"></iframe>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
setup() {
const myIframe = ref<HTMLIFrameElement>();
// 发送数据给 iframe
const sendMessage = (data: any) => {
if (myIframe.value) {
myIframe.value.contentWindow?.postMessage(data, '*');
}
};
// 接收来自 iframe 的数据
window.addEventListener('message', (event) => {
console.log('Received message from iframe:', event.data);
});
return {
myIframe,
sendMessage
};
}
});
</script>
```
在上面的代码中,我们创建了一个 `ref` 引用 `myIframe`,并在模板中将其绑定到一个 `iframe` 元素上。然后,我们定义了一个 `sendMessage` 函数,它将数据发送到 `iframe` 中。当 `iframe` 发送消息时,我们在窗口上添加了一个监听器来接收来自 `iframe` 的数据。
阅读全文
相关推荐
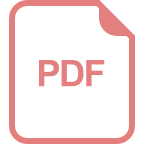








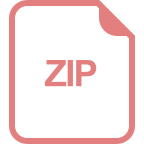
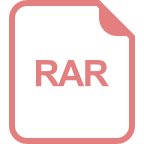
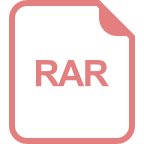


