C#实现ssh远程linux运行某个java命令,如果该命令15分钟内仍未结束则杀死该进程并执行下一条java命令
时间: 2024-10-21 21:14:56 浏览: 34
在C#中通过SSH库如SSH.NET来连接Linux服务器并执行命令,你需要完成以下几个步骤:
1. 首先,安装SSH.NET库,可以使用NuGet包管理器添加`SSH.NET`包到你的项目中。
```bash
Install-Package SSH.NET
```
2. 创建SSH客户端连接:
```csharp
using SSHParse;
using System;
using System.Net.Security;
public class SshExecutor
{
private readonly string _host;
private readonly int _port;
private readonly string _username;
private readonly string _password; // 或者使用私钥
public SshExecutor(string host, int port, string username, string password)
{
_host = host;
_port = port;
_username = username;
_password = password;
}
public void ExecuteCommandWithTimeout(string command, TimeSpan timeout)
{
using (var client = new SshClient())
{
client.HostName = _host;
client.Port = _port;
client.Username = _username;
if (!_password.IsNullOrEmpty())
{
client.Password = _password;
}
else
{
client.KeyPath = "/path/to/your/private/key"; // 使用公钥认证
}
try
{
client.Connect();
var startInfo = new ProcessStartInfo("bash", $"-c \"{command}\"");
startInfo.UseShellExecute = false;
startInfo.RedirectStandardOutput = true;
startInfo.RedirectStandardError = true;
startInfo.StandardOutputEncoding = Encoding.UTF8;
startInfo.ErrorOutputEncoding = Encoding.UTF8;
var process = new Process { StartInfo = startInfo };
process.Start();
var sw = new Stopwatch();
sw.Start();
while (sw.Elapsed <= timeout && process.HasExited == false)
{
process.StandardOutput.ReadLine(); // 检查输出以便于检查是否超时
process.StandardError.ReadLine(); // 错误输出
Thread.Sleep(1000); // 等待一段时间后再次检查
}
if (process.HasExited)
{
Console.WriteLine($"Command '{command}' finished.");
}
else
{
Console.WriteLine($"Killing timed out process for '{command}'.");
process.Kill();
}
}
catch (Exception ex)
{
Console.WriteLine($"Failed to execute command: {ex.Message}");
}
finally
{
client.Disconnect();
}
}
}
}
```
3. 调用方法并设置超时时长:
```csharp
SshExecutor executor = new SshExecutor("your.server.ip", 22, "your_username", "your_password");
executor.ExecuteCommandWithTimeout("java -jar your-jar.jar", TimeSpan.FromMinutes(15));
```
4. 如果你需要执行多个命令,可以在`ExecuteCommandWithTimeout`方法外部包装循环,并检查前一个命令的状态再执行下一个。
注意:这个示例假设你正在使用默认的SSH端口22,如果没有,需要替换相应值。另外,根据实际情况调整错误处理代码,以适应实际生产环境需求。
阅读全文
相关推荐
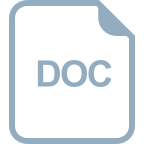
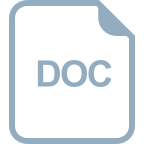
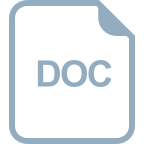
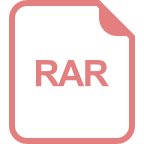
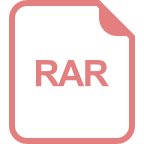
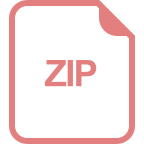
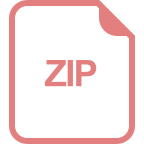
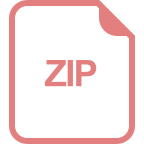
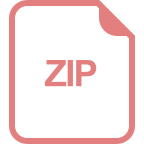
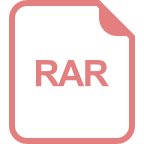
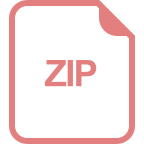
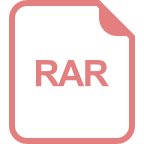
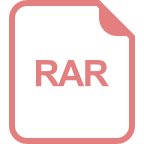
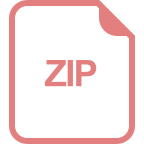
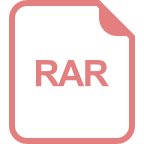
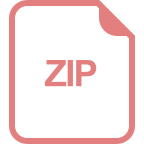
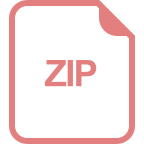
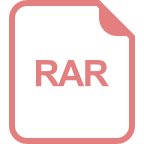
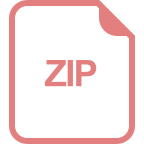