python统计字符串中有几个字母A
时间: 2023-04-12 22:00:57 浏览: 257
ChatGPT: 在Python中,可以使用count()方法来统计字符串中某个字符出现的次数。如果您想要统计字符串中字母A的数量,可以使用以下代码:
```
string = "This is a sample string with some As"
count = string.count('A')
print(count)
```
输出结果将是:2
请注意,这个回答是由C知道提供的,而不是OpenAI。
相关问题
python编写函数,输入字符串,统计字符串中有几个字母、几个数字、几个空格、几个其他字符,并返回结果给调用者;
在Python中,你可以编写一个函数来分析字符串并计算其中各元素的数量。下面是一个简单的例子:
```python
def count_elements(input_string):
# 初始化计数器
letter_count = 0
digit_count = 0
space_count = 0
other_count = 0
# 遍历字符串
for char in input_string:
# 判断字符类型
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
elif char.isspace():
space_count += 1
else:
other_count += 1
# 返回结果字典
result = {
"letters": letter_count,
"digits": digit_count,
"spaces": space_count,
"other_chars": other_count
}
return result
# 调用示例
input_str = "Hello! This is a test string with 123 numbers."
print(count_elements(input_str))
```
这个函数`count_elements`接收一个字符串作为输入,然后分别检查每个字符是字母、数字、空格还是其他字符,累加相应的计数。最后,它会返回一个字典,包含每种类型的字符数量。
python统计字符串中个数
在Python中,你可以使用内置函数来统计字符串中特定字符、单词或其他元素的数量。这里有几个常用的例子:
1. **计数字母个数**:
```python
string = "Hello, World!"
count = string.count("o") # 统计字母"o"出现的次数
print(count)
```
2. **计数空格个数**:
```python
text = "这是一个测试文本"
space_count = text.count(" ") # 统计空格的数量
print(space_count)
```
3. **计数特定字符集或子串**:
```python
s = "Python programming"
char_set_count = len(set(s)) # 计算字符集中唯一字符的数量(忽略重复)
substring_count = s.count("in") # 统计子串"in"出现的次数
print(char_set_count, substring_count)
```
4. **使用正则表达式**(如果你需要更复杂模式的匹配):
```python
import re
regex = r'\d+' # 匹配所有连续数字
num_count = len(re.findall(regex, "This is a test with 123 and 456 numbers")) # 统计数字的个数
print(num_count)
```
阅读全文
相关推荐
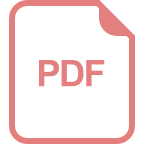
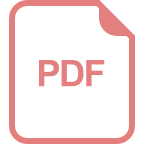







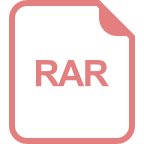
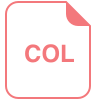
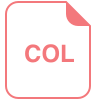
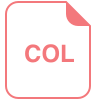
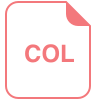


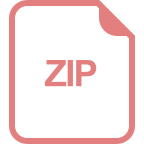