nodejs通过usb模块命令usb打印机打印
时间: 2024-09-25 22:10:12 浏览: 23
在 Node.js 中通过 `node-usb` 模块控制 USB 打印机进行打印,首先你需要确保安装了 `node-usb` 包。下面是一个基本的步骤:
1. **安装依赖**:
```bash
npm install node-usb
```
2. **初始化USB模块**:
```javascript
const usb = require('node-usb');
```
3. **查找打印机**:
使用 `findDevices` 或 `findByIds` 方法寻找特定制造商和型号的打印机。例如:
```javascript
const printerVid = 0x04b8; // 打印机Vendor ID
const printerPid = 0x0009; // 打印机Product ID
const devices = usb.findAll({ idVendor: printerVid, idProduct: printerPid });
```
4. **连接打印机**:
如果找到设备,选择其中一个并打开它:
```javascript
let device;
for (const d of devices) {
device = d;
break; // 只取第一个找到的设备,根据实际需求调整
}
if (!device) {
console.error("No printer found");
return;
}
const connection = await device.open();
```
5. **发送打印命令**:
使用 `connection.controlTransfer` 发送 ESC/POS 打印指令,比如文本、图片等。这里是一个简单的文本打印示例:
```javascript
const command = Buffer.from([0x1B, 0x2A, 0x01, 0x0D, 'Hello World!\r\n', 0x1B, 0x40]); // 打印一行文本
await connection.controlTransfer({
type: usb.constants.controlType OUT,
recipient: usb.constants.recipient DEVICE,
value: 0x00,
index: 0x00,
data: command
});
```
注意,ESC/POS 控制码是二进制数据,可能需要根据打印机的文档定制。
6. **关闭连接**:
打印完成后记得关闭连接:
```javascript
await connection.close();
```
相关推荐
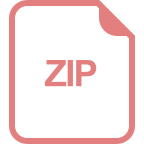
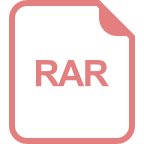














