java监听目录被上传文件
时间: 2023-10-22 14:25:00 浏览: 84
要监听目录下的文件上传,你需要使用 Java 的 WatchService 来监控文件夹中的变化,并且要检查新文件是否是上传的文件。
以下是一个简单的示例代码:
```
import java.nio.file.*;
public class FolderWatcher {
public static void main(String[] args) throws Exception {
// 获取要监控的文件夹路径
Path folderPath = Paths.get("path/to/folder");
// 创建 WatchService 对象并注册监听事件
WatchService watchService = FileSystems.getDefault().newWatchService();
folderPath.register(watchService, StandardWatchEventKinds.ENTRY_CREATE);
// 监听文件夹变化
while (true) {
WatchKey key = watchService.take();
for (WatchEvent<?> event : key.pollEvents()) {
if (event.kind() == StandardWatchEventKinds.ENTRY_CREATE) {
Path filePath = ((WatchEvent<Path>) event).context();
Path fullPath = folderPath.resolve(filePath);
if (isUploadedFile(fullPath)) {
System.out.println("New file uploaded: " + fullPath);
}
}
}
key.reset();
}
}
// 判断文件是否是上传的文件
private static boolean isUploadedFile(Path filePath) {
// TODO: 根据你的上传逻辑来判断文件是否是上传的文件
return true;
}
}
```
这个程序会不断监听指定的文件夹,如果有新增的文件出现,并且被判断为上传的文件,就会打印出文件路径。你可以将其中的 "path/to/folder" 替换成你要监控的文件夹的路径,并且根据你的上传逻辑来实现 isUploadedFile 方法。
阅读全文
相关推荐
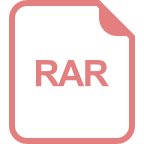
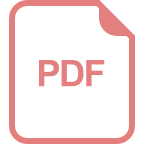
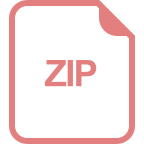
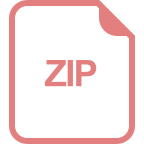
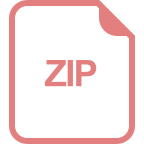
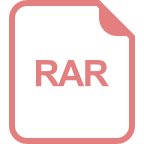
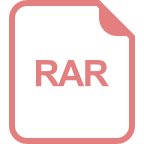
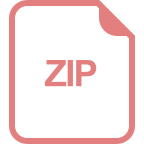
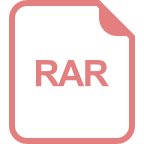
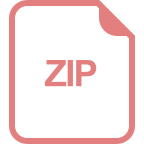
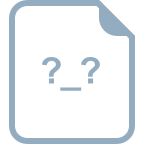
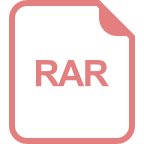
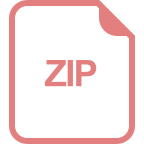
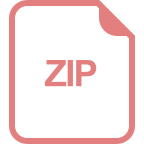
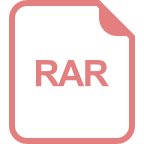
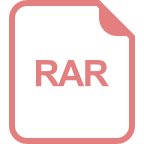
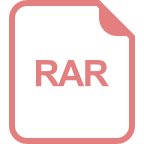
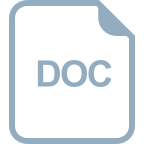