代码实现ANSI X 9.17的伪随机数产生器
时间: 2024-02-28 15:49:42 浏览: 32
ANSI X9.17是一种密码学标准,用于生成伪随机数。下面是一个使用Python实现ANSI X9.17伪随机数生成器的示例代码:
```python
import hashlib
def ANSI_X9_17_PRNG(seed, num_bytes):
key = hashlib.sha256(seed).digest()
output = b""
while len(output) < num_bytes:
key = hashlib.sha256(key).digest()
output += key
return output[:num_bytes]
seed = b"my_seed"
num_bytes = 16
random_bytes = ANSI_X9_17_PRNG(seed, num_bytes)
print("Random bytes:", random_bytes.hex())
```
在这个示例中,我们使用SHA-256哈希函数作为伪随机数生成器的基础。首先,我们将种子(seed)作为输入,使用SHA-256哈希函数生成一个密钥(key)。然后,我们将密钥作为输入再次使用SHA-256哈希函数生成新的密钥,并将其追加到输出(output)中。重复这个过程,直到输出的字节数达到所需的数量。最后,我们返回输出的前num_bytes字节作为伪随机数。
请注意,这只是一个简单的示例代码,实际使用中可能需要更复杂的实现来满足安全性要求。
相关问题
intel r hd graphics family9.17.10.4229
Intel HD Graphics Family 9.17.10.4229是英特尔的一款图形处理器驱动程序版本号。该版本的驱动程序是用来控制Intel HD Graphics系列显卡运行的软件。这些显卡通常嵌入在英特尔的处理器中,用于处理图形和视频的显示。
该驱动版本9.17.10.4229可能是在2014年发布的,所以它可能已经过时。后续版本的驱动程序往往包含更多的优化和新的功能,以提升图形处理性能和兼容性。
驱动程序的更新可以通过访问英特尔官方网站来获取。更新驱动程序可能会解决一些已知的问题和错误,并提供更好的图形性能。同时,驱动程序的更新还可以支持新的技术和功能,例如更高的分辨率、更流畅的视频播放和新的游戏效果。
安装驱动程序的过程相对简单。用户可以下载最新版本的驱动程序,并按照提示进行安装。通常需要重启计算机以使驱动程序生效。在安装过程中,可能需要解压缩文件并运行安装程序。英特尔还提供了一些工具,用于检测当前显卡驱动程序的版本和进行驱动程序的自动更新。
在使用计算机时,出现一些图形相关问题,例如画面模糊、闪烁或黑屏等,可能与旧版本的显卡驱动程序有关。这时可以尝试更新驱动程序以解决问题。
总之,Intel HD Graphics Family 9.17.10.4229是一款旧版本的显卡驱动程序,建议用户升级到最新版本以获得更好的图形性能和兼容性。
isr4300-universalk9.17.06.05.spa.bin
isr4300-universalk9.17.06.05.spa.bin 是思科路由器 ISR 4300 系列的固件版本。它是适用于大多数通用机型的固件,可提供路由器的基本功能,如数据转发、网络安全和流量管理等。此版本还可能包括一些新的功能和错误修复。因此,如果用户使用思科 ISR 4300 路由器,建议他们升级到此版本以获得最新的功能和修复。但也需要注意,在升级过程中可能会出现一些未知问题,用户需要在操作前备份好重要数据并参考思科官方文档进行操作。总之,isr4300-universalk9.17.06.05.spa.bin 是思科 ISR 4300 路由器所需的固件版本,升级后可能提供更好的网络表现和安全性。
相关推荐
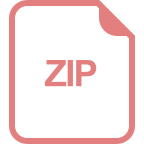











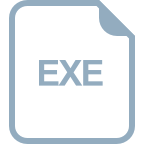