condition_variable在linux的用法
时间: 2023-09-01 15:08:55 浏览: 96
在Linux环境下,condition_variable通常与mutex一起使用,用于实现多线程之间的同步和互斥。
下面是一个简单的例子:
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <chrono>
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void work_func() {
// 模拟耗时操作
std::this_thread::sleep_for(std::chrono::milliseconds(1000));
std::cout << "work done" << std::endl;
// 通知主线程任务已完成
std::unique_lock<std::mutex> lock(mtx);
ready = true;
cv.notify_one();
}
int main() {
// 创建工作线程
std::thread work_thread(work_func);
// 等待工作线程完成工作
std::unique_lock<std::mutex> lock(mtx);
while (!ready) {
cv.wait(lock);
}
std::cout << "main thread get notified" << std::endl;
// 等待工作线程退出
work_thread.join();
return 0;
}
```
在上面的例子中,主线程等待工作线程完成工作。主线程通过condition_variable的wait()函数等待工作线程的通知。工作线程完成工作后,调用notify_one()函数通知主线程。
需要注意的是,wait()函数会自动释放锁,在接收到通知后重新获取锁。因此,在调用wait()函数前必须先获取锁。同时,在等待之前,需要通过while循环判断条件是否满足,避免虚假唤醒。
阅读全文
相关推荐
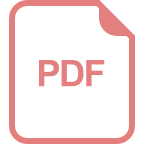
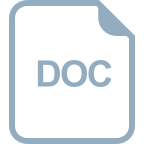
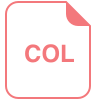
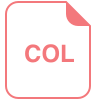
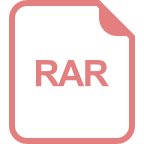
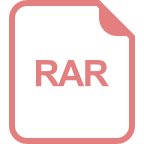
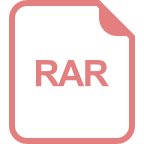
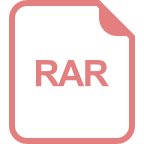
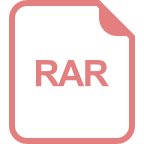
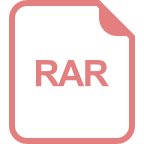
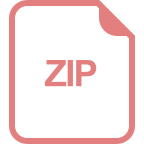
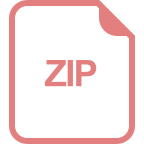
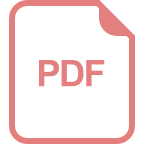
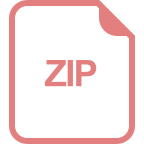
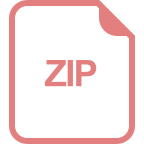
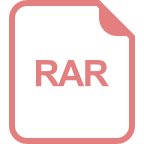
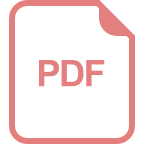