用c语言将线性表的基本操作用代码实现
时间: 2024-02-05 15:13:48 浏览: 75
以下是一个简单的线性表的基本操作的 C 语言代码实现:
```c
#define MAXSIZE 100 //线性表的最大长度
typedef struct {
int data[MAXSIZE]; //存储元素的数组
int length; //线性表的当前长度
} SqList; //顺序表类型定义
// 初始化线性表
void InitList(SqList *L) {
L->length = 0;
}
// 判断线性表是否为空
int IsEmpty(SqList L) {
return L.length == 0;
}
// 获取线性表中第i个元素的值
int GetElem(SqList L, int i) {
if (i < 1 || i > L.length) {
printf("位置不合法\n");
return -1;
}
return L.data[i - 1];
}
// 在线性表的第i个位置插入一个元素
void InsertElem(SqList *L, int i, int e) {
if (i < 1 || i > L->length + 1) {
printf("位置不合法\n");
return;
}
if (L->length == MAXSIZE) {
printf("线性表已满\n");
return;
}
for (int j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1];
}
L->data[i - 1] = e;
L->length++;
}
// 删除线性表中第i个元素
void DeleteElem(SqList *L, int i) {
if (i < 1 || i > L->length) {
printf("位置不合法\n");
return;
}
for (int j = i; j <= L->length - 1; j++) {
L->data[j - 1] = L->data[j];
}
L->length--;
}
// 获取线性表中元素的个数
int GetLength(SqList L) {
return L.length;
}
```
以上代码实现了线性表的初始化、判断是否为空、获取指定位置的元素值、在指定位置插入元素、删除指定位置的元素、获取线性表长度等基本操作。
阅读全文
相关推荐

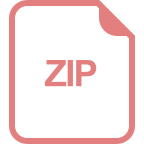
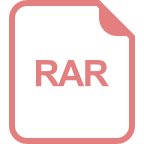








