帮我写一个自动微信聊天的JAVA程序
时间: 2023-08-31 11:41:07 浏览: 141
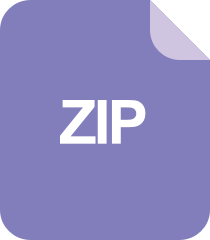
基于SpringBoot+mysql聊天微信小程序源码
### 回答1:
我可以为您提供一些有关编写自动微信聊天的JAVA程序的建议。首先,您可以使用Java WebSocket API来实现与微信服务器的连接。其次,您可以使用Java的聊天机器人框架来实现自动聊天智能。最后,您可以使用Java的图形用户界面框架来实现聊天界面。
### 回答2:
你好!要编写一个自动微信聊天的JAVA程序,首先需要了解微信的开放接口以及相关的API文档。
以下是一个简单的JAVA程序示例,用于模拟自动发送消息给微信好友并接收他们的回复:
```java
import com.alibaba.fastjson.JSONObject;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class WeChatAutoChat {
public static void main(String[] args) {
String userId = "your_user_id"; // 替换为你的用户ID
String apiKey = "your_api_key"; // 替换为你的API密钥
String apiUrl = "http://apiurl.com"; // 替换为微信API的URL
// 定义发送的消息内容
String message = "Hello, 你好!";
// 发送消息
sendWeChatMessage(apiUrl, userId, apiKey, message);
}
public static void sendWeChatMessage(String apiUrl, String userId, String apiKey, String message) {
try {
URL url = new URL(apiUrl + "/sendmessage"); // 发送消息的API接口
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.setRequestProperty("Content-Type", "application/json");
JSONObject jsonParam = new JSONObject();
jsonParam.put("userId", userId);
jsonParam.put("apiKey", apiKey);
jsonParam.put("message", message);
OutputStream os = conn.getOutputStream();
os.write(jsonParam.toString().getBytes());
os.flush();
if (conn.getResponseCode() != HttpURLConnection.HTTP_OK) {
throw new RuntimeException("Failed: HTTP error code: " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
String output;
while ((output = br.readLine()) != null) {
System.out.println(output);
}
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
该示例中,我们通过发送HTTP POST请求到微信API的发送消息接口,并传递相应的参数,包括用户ID、API密钥和发送的消息内容。程序将接收到的API回复打印到控制台。
请注意,这只是一个简单的示例,实际开发中可能需要根据微信API的具体要求进行参数设置和数据处理。
希望能对你编写自动微信聊天的JAVA程序有所帮助!如有其他问题,请随时提问。
阅读全文
相关推荐
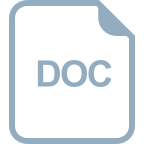
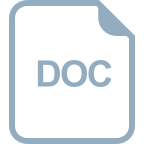
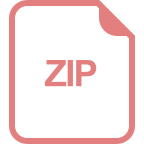

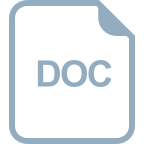
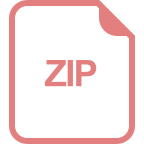
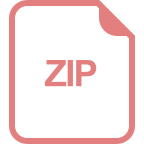
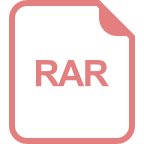
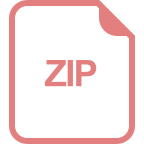
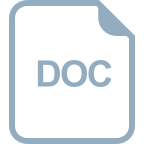
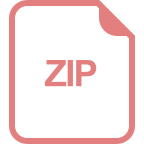
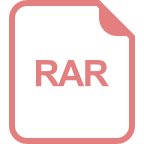
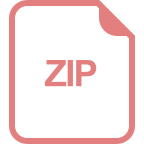
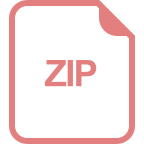
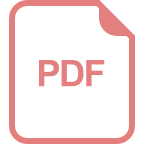
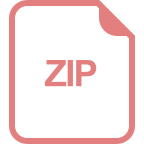
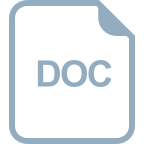
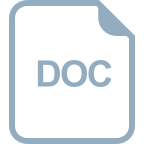