/** * Note: The returned array must be malloced, assume caller calls free(). */ int* twoSum(int* nums, int numsSize, int target,int* returnSize){ int i=0,j=0; for(i=0;i<numsSize;i++){ if(nums[i]<target){ target=target-nums[i]; for(j=i+1;j<numsSize;j++){ if(nums[j]==target){ returnSize[0]=i; returnSize[1]=j; break; } } } } return returnSize; }
时间: 2023-06-16 07:06:53 浏览: 51
这是一段实现两数之和的 C 代码。输入一个整数数组 nums,数组长度为 numsSize,以及目标值 target。函数返回一个长度为 2 的整数数组,其中包含两个元素的索引,这两个元素相加等于目标值 target。
该函数的实现过程如下:
1. 遍历整个数组 nums。
2. 对于每个元素 nums[i],计算目标值 target 减去该元素的差值(即 diff = target - nums[i])。
3. 在剩下的元素中找到一个值等于 diff 的元素,它的索引为 j。
4. 返回数组 [i, j]。
需要注意的是,该函数中的 returnSize 是一个指针,用于返回数组的长度。在函数内部,分配了一个长度为 2 的整数数组,用于存储返回结果。最后将该数组的指针返回即可。
相关问题
Note: The returned array must be malloced, assume caller calls free()
on the result array.
To allocate memory dynamically in C, we use the malloc() function. The syntax for using malloc() is as follows:
```
ptr = (castType*) malloc(size);
```
Here, ptr is a pointer of type castType. The malloc() function allocates a block of size bytes of memory, and returns a pointer to the first byte of the block. We can then use this pointer to access the block of memory.
In the context of the given problem, we need to allocate memory for a new array of size n, where n is the length of the input array. We can use malloc() to do this as follows:
```
int* result = (int*) malloc(n * sizeof(int));
```
Here, we first cast the pointer returned by malloc() to an int pointer, as we are allocating memory for an array of integers. We then multiply the size of an int (in bytes) by n, to get the total number of bytes to allocate. Finally, we assign the pointer returned by malloc() to the result variable.
The complete code for the function is as follows:
```
int* runningSum(int* nums, int numsSize, int* returnSize){
int* result = (int*) malloc(numsSize * sizeof(int));
int sum = 0;
for (int i = 0; i < numsSize; i++) {
sum += nums[i];
result[i] = sum;
}
*returnSize = numsSize;
return result;
}
```
Here, we first allocate memory for the result array using malloc(). We then iterate through the input array, computing the running sum and storing it in the result array. Finally, we set the value of returnSize to numsSize (as the length of the result array is equal to the length of the input array), and return the result array.
/** * Note: The returned array must be malloced, assume caller calls free(). / int twoSum(int* nums, int numsSize, int target,int* returnSize){ int i=0,j=0; for(i=0;i<numsSize;i++){ if(nums[i]<target){ target=target-nums[i]; for(j=i+1;j<numsSize;j++){ if(nums[j]==target){ returnSize[0]=i; returnSize[1]=j; break; } } } } return returnSize; }
这是一个 C 语言的函数,实现了在给定整型数组 nums 中找到两个数,使得它们的和等于目标值 target。函数的返回值是一个指向整型数组的指针,该数组包含两个元素,分别是找到的两个数在 nums 中的下标。同时,该函数还接收一个指向整型变量的指针,用于返回数组的长度,也就是 2。
函数的实现过程为,首先对 nums 数组进行一次遍历,每次取出一个数,计算该数与 target 的差值,并将该差值作为新的目标值。然后再对 nums 数组进行一次遍历,查找是否存在一个数与目标值相等,如果存在,则将该数的下标赋值给数组的第二个元素,并将前面取出的数的下标赋值给数组的第一个元素。最后返回数组指针即可。
需要注意的是,该函数是在调用函数内部申请了一个数组,因此在调用函数结束后需要手动释放该数组。可以使用 free() 函数来释放该数组。
另外,该函数的时间复杂度为 O(n^2),不适用于大规模的数据。可以使用哈希表等数据结构来优化算法,将时间复杂度降为 O(n)。
相关推荐
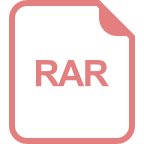
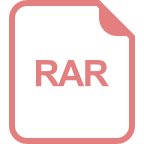
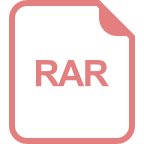






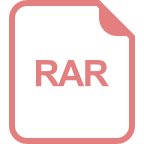
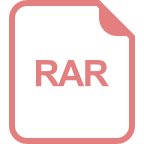
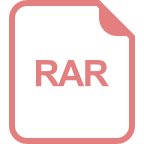
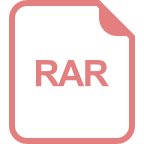
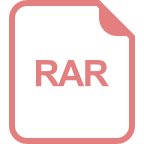
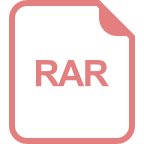