使用pyspark mllib sparkcontext,文件每一行第一列为整数0或1,后面为浮点数。要求对数据集进行划分,使用rdd类型。使用SVM进行训练,最后进行评估。要求有中间过程的输出
时间: 2023-11-28 08:50:22 浏览: 65
首先,我们需要先读取文件并将其转换为RDD类型:
```python
from pyspark import SparkContext, SparkConf
from pyspark.mllib.regression import LabeledPoint
from pyspark.mllib.classification import SVMWithSGD
from pyspark.mllib.evaluation import BinaryClassificationMetrics
conf = SparkConf().setAppName("SVM Example")
sc = SparkContext(conf=conf)
data = sc.textFile("file.txt")
parsedData = data.map(lambda line: line.split()).map(lambda x: LabeledPoint(x[0], x[1:]))
```
接下来,我们将数据集分为训练集和测试集:
```python
# Split data into training (60%) and test (40%)
trainingData, testData = parsedData.randomSplit([0.6, 0.4], seed=11L)
```
然后,我们使用SVMWithSGD算法进行模型训练:
```python
# Train SVM model
model = SVMWithSGD.train(trainingData)
```
接下来,我们对测试集进行预测并计算模型的性能指标:
```python
# Predict on test data and compute metrics
predictions = model.predict(testData.map(lambda x: x.features))
labelsAndPredictions = testData.map(lambda lp: lp.label).zip(predictions)
metrics = BinaryClassificationMetrics(labelsAndPredictions)
# Print model performance metrics
print("Area under ROC = %s" % metrics.areaUnderROC)
```
以上就是使用pyspark mllib sparkcontext对数据集进行划分,使用SVM进行训练,最后进行评估的基本过程。在上述过程中,我们使用了LabeledPoint对象来表示数据点,并使用SVMWithSGD算法进行模型训练。最后,我们使用BinaryClassificationMetrics类计算模型的性能指标。
阅读全文
相关推荐
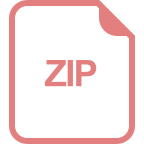
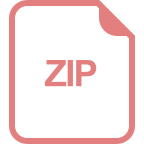
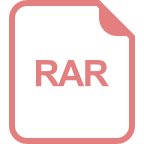
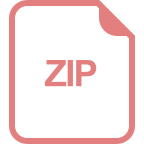
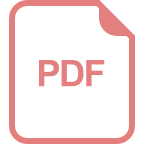
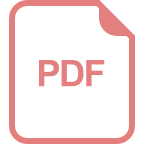
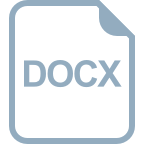
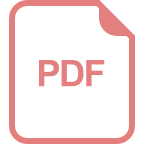
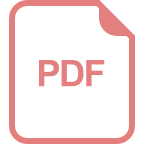
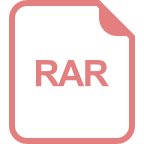
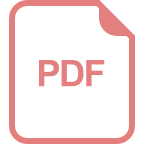


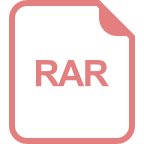