用Verilog写一个没有读延时的fifo
时间: 2024-05-09 10:19:57 浏览: 148
以下是一个简单的Verilog代码,实现了一个没有读延时的fifo:
```verilog
module fifo (
input clk,
input reset,
input write_en,
input [7:0] write_data,
output reg empty,
output reg full,
output reg [7:0] read_data,
input read_en
);
parameter WIDTH = 8;
parameter DEPTH = 16;
reg [WIDTH-1:0] mem [0:DEPTH-1];
reg [4:0] head = 0;
reg [4:0] tail = 0;
reg [4:0] count = 0;
always @(posedge clk) begin
if (reset) begin
head <= 0;
tail <= 0;
count <= 0;
empty <= 1;
full <= 0;
end else begin
if (write_en && !full) begin
mem[head] <= write_data;
head <= head + 1;
count <= count + 1;
empty <= 0;
if (count == DEPTH) begin
full <= 1;
end
end
if (read_en && !empty) begin
read_data <= mem[tail];
tail <= tail + 1;
count <= count - 1;
full <= 0;
if (count == 1) begin
empty <= 1;
end
end
end
end
endmodule
```
该代码使用一个双端队列(circular queue)实现了一个FIFO。双端队列具有一个头指针(head)和一个尾指针(tail),写入数据时将数据存储在头指针对应的位置,头指针向后移动一位,读取数据时将尾指针对应的数据读出,尾指针向后移动一位。当头指针和尾指针相遇时,可以通过将头指针或尾指针移回队列的起始位置来实现循环队列的效果。队列的状态包括当前队列中的元素数量(count)和队列是否为空或已满(empty和full)。在写入或读取数据时,需要检查队列是否已满或为空,以避免数据覆盖或读取错误。
需要注意的是,这个FIFO没有读延时,因此读取到的数据是上一个时钟周期写入的数据。如果需要读延时,可以在读取数据时将数据暂存,并在下一个时钟周期输出。
阅读全文
相关推荐
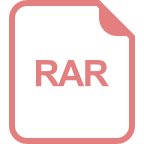
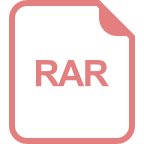
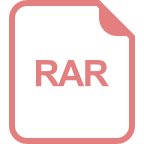
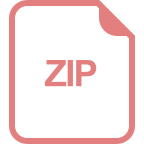
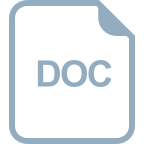




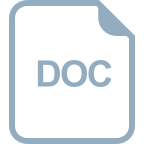
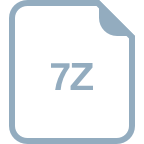
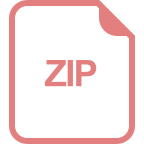
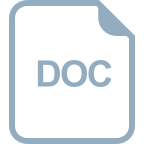
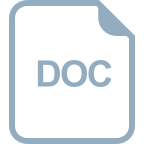
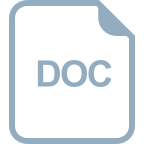