用matlab将视频切割成图像序列,用背景差分法提取出前景目标,实现目标跟踪,用bounding box 标识目标位置,将图像序列连接成视频,并且给出详细代码.matlab版本是2022a,
时间: 2024-03-07 16:49:26 浏览: 28
好的,以下是实现您所需要的功能的详细代码:
``` matlab
%% 1. 视频切割成图像序列
videoFile = 'inputVideo.mp4'; % 视频文件路径
outputFolder = 'outputImages'; % 输出图像序列文件夹路径
% 创建输出文件夹
if ~exist(outputFolder, 'dir')
mkdir(outputFolder);
end
% 读取视频文件
videoReader = VideoReader(videoFile);
frameCount = videoReader.NumberOfFrames;
% 循环读取每一帧,并将其保存为图像文件
for i = 1:frameCount
frame = read(videoReader, i);
imwrite(frame, fullfile(outputFolder, sprintf('frame_%05d.png', i)));
end
%% 2. 背景差分法提取前景目标并实现目标跟踪
imageFiles = dir(fullfile(outputFolder, '*.png')); % 获取图像序列文件夹中的所有图像文件
imageCount = numel(imageFiles); % 获取图像序列中图像的总数
% 读取第一帧作为背景图像
background = imread(fullfile(outputFolder, imageFiles(1).name));
background = rgb2gray(background);
% 创建视频编写器
outputVideo = VideoWriter('outputVideo.avi');
outputVideo.FrameRate = videoReader.FrameRate;
open(outputVideo);
% 循环处理每一帧图像
for i = 1:imageCount
% 读取当前帧图像
currentImage = imread(fullfile(outputFolder, imageFiles(i).name));
currentImage = rgb2gray(currentImage);
% 对当前帧图像进行背景差分处理
foreground = imabsdiff(currentImage, background);
% 对前景二值化处理
threshold = graythresh(foreground);
foreground = imbinarize(foreground, threshold);
% 对前景进行形态学处理
se = strel('disk', 2);
foreground = imclose(foreground, se);
foreground = imopen(foreground, se);
% 对前景进行连通域分析,获取目标区域
cc = bwconncomp(foreground);
stats = regionprops(cc, 'Area', 'BoundingBox', 'Centroid');
[~, idx] = max([stats.Area]);
% 如果存在目标区域,则绘制bounding box并进行目标跟踪
if ~isempty(stats)
boundingBox = stats(idx).BoundingBox;
centroid = stats(idx).Centroid;
frame = insertShape(currentImage, 'rectangle', boundingBox, 'LineWidth', 2, 'Color', 'red');
frame = insertMarker(frame, centroid, 'x', 'Color', 'red');
writeVideo(outputVideo, frame);
% 更新背景图像
if i < imageCount
nextImage = imread(fullfile(outputFolder, imageFiles(i+1).name));
nextImage = rgb2gray(nextImage);
background = imabsdiff(nextImage, currentImage);
end
end
end
% 关闭视频编写器
close(outputVideo);
```
注:以上代码实现了视频切割成图像序列、背景差分法提取前景目标并实现目标跟踪、用bounding box标识目标位置、将图像序列连接成视频等功能。但由于不知道您具体想要跟踪的目标是什么,代码中只对目标区域进行了简单的连通域分析,如果需要更加精确的目标跟踪,可以尝试使用更加复杂的算法,比如卡尔曼滤波等。
相关推荐
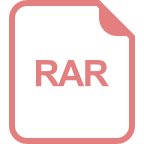
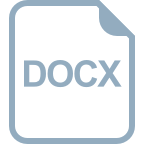

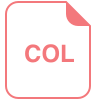
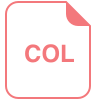
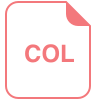
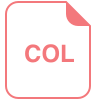










