python 假设我们有一个字典,其中包含多个字典,每个字典代表一个人的信息,往字典中继续记录一个的信息,并将结果生成excel
时间: 2023-12-29 15:54:30 浏览: 26
可以使用 python 的 pandas 库来实现这个功能。
首先,我们需要创建一个包含多个字典的字典,例如:
```python
people = {
'person1': {'name': 'Alice', 'age': 25, 'gender': 'Female'},
'person2': {'name': 'Bob', 'age': 30, 'gender': 'Male'},
'person3': {'name': 'Charlie', 'age': 35, 'gender': 'Male'}
}
```
然后,我们可以使用 pandas 的 DataFrame 类来将字典转换成数据框,并添加新的一行数据:
```python
import pandas as pd
# 转换字典为数据框
df = pd.DataFrame.from_dict(people, orient='index')
# 添加新的一行数据
df.loc['person4'] = {'name': 'David', 'age': 40, 'gender': 'Male'}
# 将数据框保存为excel文件
df.to_excel('people.xlsx')
```
这样,我们就可以将包含多个字典的字典转换成数据框,并将新的一行数据添加到其中,最后将结果保存为excel文件。
相关问题
python 字典包含多个字典演示
假设我们有一个字典,其中包含多个字典,每个字典代表一个人的信息:
```python
people = {
'person1': {'name': 'Alice', 'age': 25, 'city': 'NY'},
'person2': {'name': 'Bob', 'age': 30, 'city': 'LA'},
'person3': {'name': 'Charlie', 'age': 35, 'city': 'SF'}
}
```
我们可以使用嵌套的字典访问这些信息:
```python
print(people['person1']['name']) # 输出 'Alice'
print(people['person2']['age']) # 输出 30
print(people['person3']['city']) # 输出 'SF'
```
我们也可以使用循环遍历每个人的信息:
```python
for person, info in people.items():
print(f"{person}: {info['name']} is {info['age']} years old and lives in {info['city']}.")
```
输出:
```
person1: Alice is 25 years old and lives in NY.
person2: Bob is 30 years old and lives in LA.
person3: Charlie is 35 years old and lives in SF.
```
用python写一个函数将字典中value值列表中的每个值与字典中的key值重新生成一个字典
可以参考以下的Python代码实现:
```python
def generate_dict(d):
new_dict = {}
for key, values in d.items():
for value in values:
new_dict[value] = key
return new_dict
```
其中,参数 `d` 是要处理的字典。函数中的循环遍历每个键值对,然后再遍历其对应的值列表,将每个值与当前的键组合成一个新的键值对,最后将所有新的键值对组成一个新的字典并返回。注意,如果原来的字典中有重复的值,那么最终生成的新字典中将只保留最后一个值对应的键。
阅读全文
相关推荐
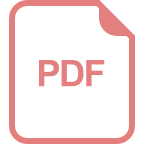
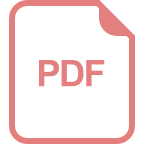













